阈值分割
灰度图
import cv2 as cv
import numpy as np
import os
import base_function as bf
sourceImg = cv.imread("static/26.png")
grayImg = cv.cvtColor(sourceImg,cv.COLOR_BGR2GRAY)
bf.cv_show("grayImg",grayImg)
效果
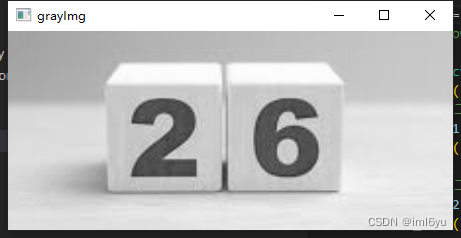
二值化
ret,thresh1 = cv.threshold(grayImg,100,255,cv.THRESH_BINARY)
bf.cv_show("THRESH_BINARY",thresh1)
效果
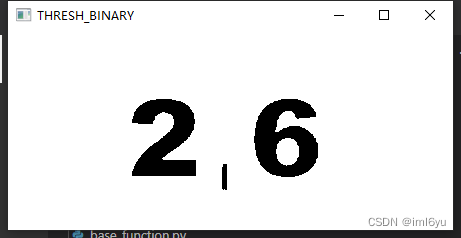
二值化取反
ret,thresh2 = cv.threshold(grayImg,100,255,cv.THRESH_BINARY_INV)
bf.cv_show("THRESH_BINARY_INV",thresh2)
效果
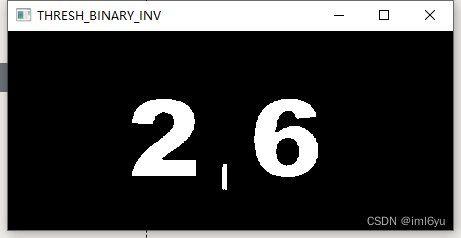
截取
ret,thresh3 = cv.threshold(grayImg,100,0,cv.THRESH_TRUNC)
bf.cv_show("THRESH_TRUNC",thresh3)
效果
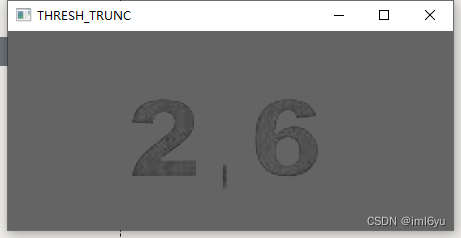
TOZERO
ret,thresh4 = cv.threshold(grayImg,100,255,cv.THRESH_TOZERO)
bf.cv_show("THRESH_TRUNC",thresh4)
效果
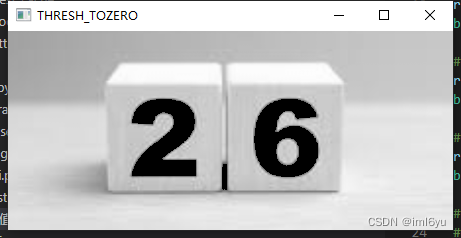
TOZERO取反
ret,thresh4 = cv.threshold(grayImg,100,255,cv.THRESH_TOZERO_INV)
bf.cv_show("THRESH_TRUNC",thresh4)
效果
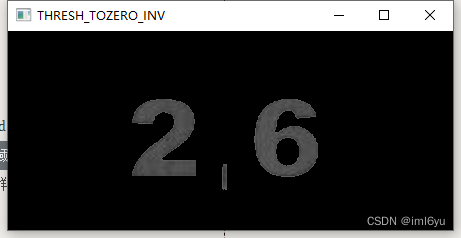
滤波
均值滤波
from math import fabs
import cv2 as cv
import numpy as np
import base_function as bf
source = cv.imread("static/1.png")
cv.imshow("source",source)
cv.waitKey(1000)
cv.destroyAllWindows()
blur = cv.blur(source,(5,5))
bf.cv_show("blur",blur)
高斯滤波
gus = cv.GaussianBlur(source,(5,5),5)
bf.cv_show("gus",gus)
中值滤波
middle = cv.medianBlur(source,5)
bf.cv_show("middle",middle)
图像拼接
简单的横向和纵向拼接
all = cv.hconcat([blur,gus,middle])
bf.cv_show("all",all)
allv = cv.vconcat([blur,gus,middle])
bf.cv_show("allv",allv)
效果(三幅图片分别是均值滤波,高斯滤波,中值滤波)
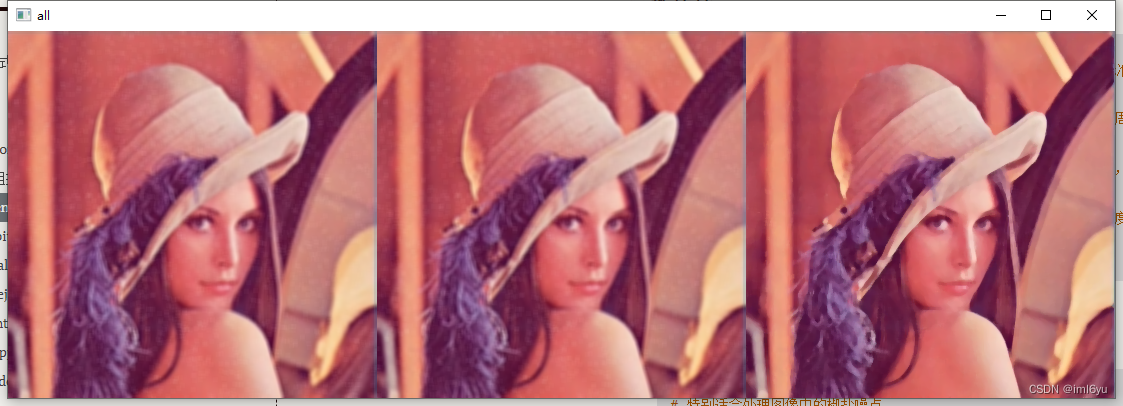
腐蚀与膨胀
import cv2 as cv
import base_function as bf
import numpy as np
position = (10, 50)
font = cv.FONT_HERSHEY_SIMPLEX
scale = 1
color = (255, 255, 255)
thickness = 2
source = cv.imread("static/26.png")
gray = cv.cvtColor(source,cv.COLOR_BGR2GRAY)
kernel = np.ones((7,7),np.uint8)
ret,binary = cv.threshold(gray,100,255,cv.THRESH_BINARY_INV)
erode = cv.erode(binary,kernel,iterations=1)
dilate = cv.dilate(erode,kernel,iterations=1)
open = cv.morphologyEx(binary,cv.MORPH_OPEN,kernel)
close = cv.morphologyEx(binary,cv.MORPH_CLOSE,kernel)
cv.putText(gray,"gray",position,font,scale,color,thickness)
cv.putText(binary,"binary",position,font,scale,color,thickness)
cv.putText(erode,"erode",position,font,scale,color,thickness)
cv.putText(dilate,"dilate",position,font,scale,color,thickness)
cv.putText(open,"open",position,font,scale,color,thickness)
cv.putText(close,"close",position,font,scale,color,thickness)
all =np.vstack((np.hstack((gray,binary,erode)) ,np.hstack((dilate,open,close))))
bf.cv_show("all",all)
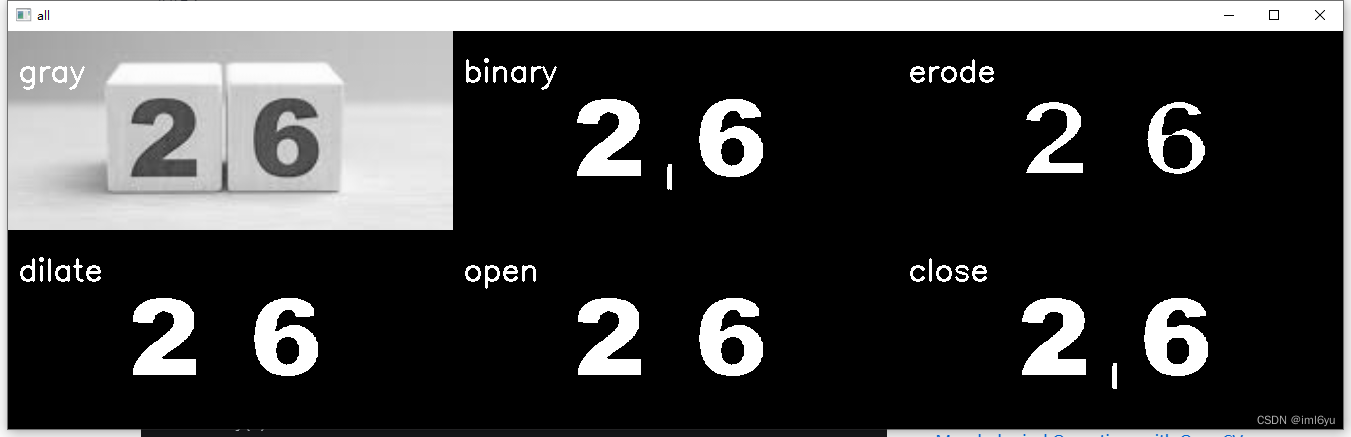