JAVA小游戏“飞翔的小鸟”
发布时间:2023年12月27日
一步是创建项目?项目名自拟
第二步创建个包名?来规范class
再创建一个包? 来存储照片
如下: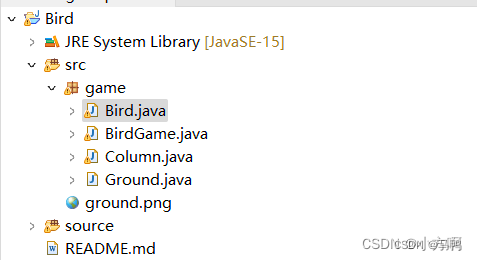
代码如下:
- package game;
import java.awt.*;
import javax.swing.*;
import javax.imageio.ImageIO;
?
public class Bird {
?
?? ?Image image;
?? ?int x,y;
?? ?int width,height;
?? ?int size;
?? ?
?? ?double g;
?? ?
?? ?double t;
?? ?
?? ?double v0;
?? ?
?? ?double speed;
?? ?
?? ?double s;
?? ?
?? ?double alpha;
?? ?
?? ?//?
?? ?Image[] images;
?? ?
?? ?int index;
?? ?
?? ?public Bird() throws Exception
?? ?{
?? ??? ?image=new ImageIcon("source/0.png").getImage();
?? ??? ?width = image.getWidth(null);
?? ??? ?height = image.getHeight(null);
?? ??? ?x=132;
?? ??? ?y=280;
?? ??? ?size=40;
?? ?
?? ??? ?g=4;
?? ??? ?v0=20;
?? ??? ?t=0.25;
?? ??? ?speed=v0;
?? ??? ?s=0;
?? ??? ?
?? ??? ?alpha=0;
?? ??? ?
?? ??? ?
?? ??? ?images=new Image[8];
?? ??? ?
?? ??? ?for(int i=0;i<8;i++)
?? ??? ?{
?? ??? ??? ?images[i]=new ImageIcon("source/"+i+".png").getImage();
?? ??? ?}
?? ??? ?index=0;
?? ??? ?
?? ?}
?? ?
?? ?
?? ?public void fly()
?? ?{
?? ??? ?index++;
?? ??? ?image=images[(index/12)%8];
?? ?}
?? ?
?? ?
?? ?public void step()
?? ?{
?? ??? ?double v0=speed;
?? ??? ?
?? ??? ?s=v0*t+g*t*t/2;
?? ??? ?
?? ??? ?y=y-(int)s;
?? ??? ?
?? ??? ?double v=v0-g*t;
?? ??? ?speed =v;
?? ??? ?
?? ??? ?alpha=Math.atan(s/8);
?? ??? ?
?? ?}
?? ?
?? ?
?? ?public void flappy()
?? ?{
?? ??? ?
?? ??? ?speed=v0;
?? ?}
?? ?
?? ?
?? ?public boolean hit(Ground ground)
?? ?{
?? ??? ?boolean hit =y+size/2>ground.y;
?? ??? ?if(hit)
?? ??? ?{
?? ??? ??? ?y=ground.y-size/2;
?? ??? ??? ?alpha=Math.PI/2;
?? ??? ?}
?? ??? ?return hit;
?? ?}
?? ?
?? ?
?? ?public boolean hit(Column column)
?? ?{
?? ??? ?
?? ??? ?if(x>column.x-column.width/2-size/2&&x<column.x+column.width/2+size/2)
?? ??? ?{
?? ??? ??? ?if(y>column.y-column.gap/2+size/2&&y<column.y+column.gap/2-size/2) return false;
?? ??? ??? ?return true;
?? ??? ?}
?? ??? ?return false;
?? ?}
}package game;
?
import javax.imageio.ImageIO;
import java.util.*;
?
import javax.swing.*;
?
import java.awt.Graphics;
import java.awt.event.MouseAdapter;
import java.awt.event.*;
import java.awt.image.BufferedImage;
import javax.imageio.*;
import java.awt.*;
?
public class BirdGame extends JPanel {
?? ?
?? ?
?? ?Image background;
?? ?Image startImage;
?? ?Image overImage;
?? ?Ground ground;//����
?? ?Column column1,column2;
?? ?Bird bird;
?? ?int score;
?? ?int state;//??
?? ?//??����
?? ?public static final int START=0;
?? ?public static final int RUNNING=1;
?? ?public static final int GAME_OVER=2;
?? ?
?? ?public BirdGame() throws Exception
?? ?{
?? ??? ?background = new ImageIcon("./source/bg.png").getImage();
?? ??? ?startImage = new ImageIcon("./source/start.png").getImage();
?? ??? ?overImage=new ImageIcon("./source/gameover.png").getImage();
?? ?//??
?? ??? ?ground=new Ground();
?? ??? ?column1=new Column(1);
?? ??? ?column2=new Column(2);
?? ??? ?bird=new Bird();
?? ??? ?score=0;
?? ??? ?state=0;
?? ?}
?? ?
?? ?public void paint(Graphics g)
?? ?{
?
?? ??? ?g.drawImage(background, 0, 0,null);
?? ??? ?
?? ??? ?g.drawImage(ground.image, ground.x, ground.y, null);
?? ?
?? ??? ?g.drawImage(column1.image,column1.x-column1.width/2,column1.y-column1.height/2,null);
?? ??? ?g.drawImage(column2.image,column2.x-column2.width/2,column2.y-column2.height/2,null);
?? ?
?? ??? ?Graphics2D g2=(Graphics2D) g;
?? ??? ?g2.rotate(-bird.alpha,bird.x,bird.y);
?? ??? ?g.drawImage(bird.image,bird.x-bird.width/2,bird.y-bird.height/2,null);
?? ??? ?g2.rotate(bird.alpha,bird.x,bird.y);
?? ??? ?
?? ??? ?Font f=new Font(Font.SANS_SERIF,Font.BOLD,40);
?? ??? ?g.setFont(f);
?? ??? ?g.drawString(""+score, 40, 60);
?? ??? ?g.setColor(Color.WHITE);
?? ??? ?g.drawString(""+score,40-3, 60-3);
?? ??? ?
?? ??? ?switch(state)
?? ??? ?{
?? ??? ?case START:
?? ??? ??? ?g.drawImage(startImage, 0, 0, null);
?? ??? ??? ?break;
?? ??? ?case GAME_OVER:
?? ??? ??? ?g.drawImage(overImage, 0, 0, null);
?? ??? ??? ?break;
?? ??? ?}
?? ?}
?? ?
?? ?public void action() throws Exception
?? ?{
?? ??? ?
?? ??? ?MouseListener l=new MouseAdapter()
?? ??? ?{
?? ??? ??? ?public void mousePressed(MouseEvent e)
?? ??? ??? ?{
?? ??? ??? ??? ?try {
?? ??? ??? ??? ??? ?switch(state) {
?? ??? ??? ??? ??? ?case START:
?? ??? ??? ??? ??? ??? ?//??
?? ??? ??? ??? ??? ??? ?state=RUNNING;
?? ??? ??? ??? ??? ??? ?break;
?? ??? ??? ??? ??? ?case RUNNING:
?? ??? ??? ??? ??? ??? ?
?? ??? ??? ??? ??? ??? ?bird.flappy();
?? ??? ??? ??? ??? ??? ?break;
?? ??? ??? ??? ??? ?case GAME_OVER:
?? ??? ??? ??? ??? ??? ?
?? ??? ??? ??? ??? ??? ?column1=new Column(1);
?? ??? ??? ??? ??? ??? ?column2=new Column(2);
?? ??? ??? ??? ??? ??? ?bird=new Bird();
?? ??? ??? ??? ??? ??? ?score=0;
?? ??? ??? ??? ??? ??? ?state=START;
?? ??? ??? ??? ??? ??? ?break;
?? ??? ??? ??? ??? ?}
?? ??? ??? ??? ?}
?? ??? ??? ??? ?catch (Exception ex)
?? ??? ??? ??? ?{
?? ??? ??? ??? ??? ?ex.printStackTrace();
?? ??? ??? ??? ?}
?? ??? ??? ?}
?? ??? ??? ?
?? ??? ?};
?? ??? ?addMouseListener(l);
?? ??? ?while(true)
?? ??? ?{
?? ??? ??? ?switch(state)
?? ??? ??? ?{
?? ??? ??? ?case START:
?? ??? ??? ??? ?bird.fly();
?? ??? ??? ??? ?ground.step();
?? ??? ??? ??? ?break;
?? ??? ??? ?case RUNNING:
?? ??? ??? ??? ?ground.step();
?? ??? ??? ??? ?column1.step();
?? ??? ??? ??? ?column2.step();
?? ??? ??? ??? ?bird.fly();
?? ??? ??? ??? ?bird.step();
?
?? ??? ??? ??? ?score++;
?? ??? ??? ??? ?//
?? ??? ??? ??? ?if(bird.hit(ground)||bird.hit(column1)||bird.hit(column2))
?? ??? ??? ??? ?{
?? ??? ??? ??? ??? ?state=GAME_OVER;
?? ??? ??? ??? ?}
?? ??? ??? ??? ?break;
?? ??? ??? ?}?? ?
?? ??? ??? ?
?? ??? ??? ?Thread.sleep(1000/60);
?? ??? ??? ?repaint();
?? ??? ?}
?? ?}
?? ?
?? ?
?? ?
?? ?public static void main(String[] args) throws Exception
?? ?{
?? ??? ?
?? ??? ?JFrame frame=new JFrame();
?? ??? ?BirdGame game=new BirdGame();
?? ??? ?frame.add(game);
?? ??? ?frame.setSize(440,670);
?? ??? ?frame.setLocationRelativeTo(null);
?? ??? ?frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
?? ??? ?frame.setVisible(true);
?? ??? ?game.action();
?? ?}
?? ?
}package game;
?
import javax.swing.*;
import java.awt.*;
?
public class Ground {
?
?? ?Image image;
?? ?
?? ?int x,y;
?? ?
?? ?int width,height;
?? ?
?? ?
?? ?public Ground() throws Exception
?? ?{
?? ??? ?image =new ImageIcon("source/ground.png").getImage();
?? ??? ?width=image.getWidth(null);
?? ??? ?height=image.getHeight(null);
?? ??? ?x=0;
?? ??? ?y=500;
?? ?}
?? ?
?? ?
?? ?public void step()
?? ?{
?? ??? ?x-=4;
?? ??? ?if(x<=-109)
?? ??? ?{
?? ??? ??? ?x=0;
?? ??? ?}
?? ?}
?? ?
?? ?
}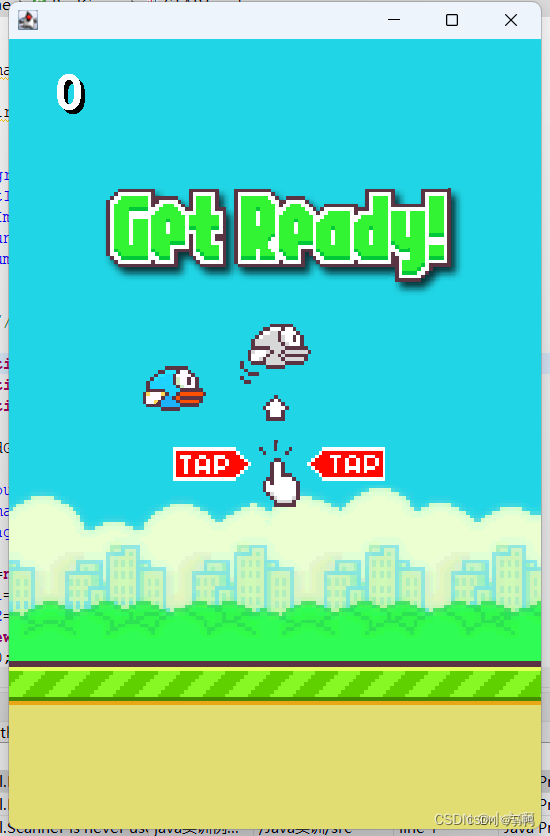
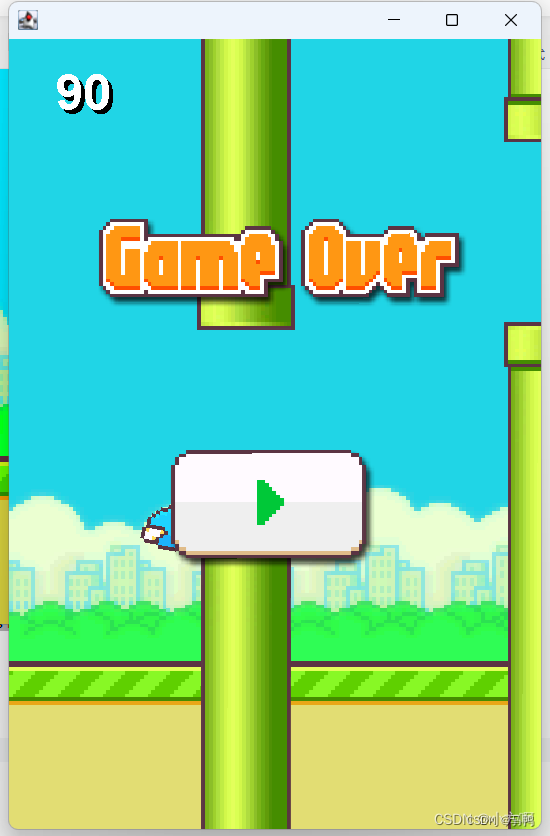
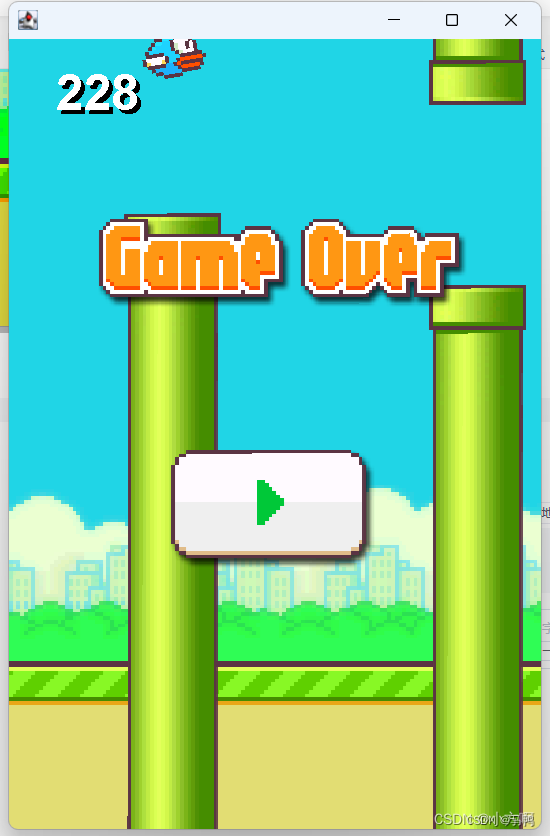
文章来源:https://blog.csdn.net/2302_76551984/article/details/135213039
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。
如若内容造成侵权/违法违规/事实不符,请联系我的编程经验分享网邮箱:chenni525@qq.com进行投诉反馈,一经查实,立即删除!