oled的上方四个接口是IIC总线 通过IIC总线可以进行数据的传输 在OLED模块背后有一个芯片叫做SSD1306 这个芯片内部有1024个字节的RAM 对应到右边的小屏幕上就有1024个字节 一个字节八个bit位 每一个bit位就对应着一个小点 我们只需要往oled的RAM上写入数据就可以显示对应的字符和图片
在单片机内部开一块buffer 也是1024kb的对应着oled屏幕 相当于一块画布 然后可以调用pal库的接口对oled画线等图案
初始化屏幕就是 oled屏幕内部有一个芯片 芯片有一个SA0引脚 如果接地就为0 如果接高电平就为1
SA0接地为0 从机地址为0111100 接高电平就为0111101 我们的oled芯片是接地的所以SA0传入的值为RESET (0)
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
while(1)
{
}
}
在X和Y的方向上移动光标 就是X和Y坐标同时变化
画笔和画刷
如要画123和一个苹果 就是先画一个矩形 画笔和画刷都设置为白色就得到一个蓝色背景的矩形
然后绘画数字123 就是用黑色的画笔 透明的画刷 (这样就不会挡住后面的背景色了) 绘画苹果也是设置黑色画笔 透明画刷
画点
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
PAL_OLED_Clear(&holed);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,3);
PAL_OLED_SetCursor(&holed,29,32);
PAL_OLED_DrawDot(&holed);
uint32_t i;
for(i=1;i<8;i++)
{
PAL_OLED_MoveCursorX(&holed,10);
PAL_OLED_DrawDot(&holed);
}
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}
画线
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
// PAL_OLED_Clear(&holed);
// PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,3);
// PAL_OLED_SetCursor(&holed,29,32);
// PAL_OLED_DrawDot(&holed);
// uint32_t i;
//
// for(i=1;i<8;i++)
// {
// PAL_OLED_MoveCursorX(&holed,10);
// PAL_OLED_DrawDot(&holed);
// }
//
// PAL_OLED_SendBuffer(&holed);
PAL_OLED_Clear(&holed);
PAL_OLED_SetCursor(&holed,17,20);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,1);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,2);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,3);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,4);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
// PAL_OLED_Clear(&holed);
// PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,3);
// PAL_OLED_SetCursor(&holed,29,32);
// PAL_OLED_DrawDot(&holed);
// uint32_t i;
//
// for(i=1;i<8;i++)
// {
// PAL_OLED_MoveCursorX(&holed,10);
// PAL_OLED_DrawDot(&holed);
// }
//
// PAL_OLED_SendBuffer(&holed);
PAL_OLED_Clear(&holed);
PAL_OLED_SetCursor(&holed,17,20);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,1);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,2);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,3);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_MoveCursorY(&holed,8);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,4);
PAL_OLED_DrawLine(&holed,PAL_OLED_GetCursorX(&holed) + 45,PAL_OLED_GetCursorY(&holed));
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE,1);
PAL_OLED_SetCursor(&holed,91,10);
PAL_OLED_LineTo(&holed,87,24);
PAL_OLED_LineTo(&holed,72,24);
PAL_OLED_LineTo(&holed,84,33);
PAL_OLED_LineTo(&holed,80,48);
PAL_OLED_LineTo(&holed,91,38);
PAL_OLED_LineTo(&holed,101,48);
PAL_OLED_LineTo(&holed,97,33);
PAL_OLED_LineTo(&holed,110,24);
PAL_OLED_LineTo(&holed,95,24);
PAL_OLED_LineTo(&holed,91,10);
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}
画矩形和画圆
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
PAL_OLED_Clear(&holed);
PAL_OLED_SetCursor(&holed,0,0);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE ,1);
PAL_OLED_SetBrush(&holed,BRUSH_WHITE);//设置背景颜色
PAL_OLED_DrawRect(&holed,PAL_OLED_GetScreenWidth(&holed),PAL_OLED_GetScreenHeight(&holed));
PAL_OLED_SetPen(&holed,OLED_COLOR_BLACK ,1);
PAL_OLED_SetBrush(&holed,BRUSH_TRANSPARENT);
PAL_OLED_SetCursor(&holed,20,20);
PAL_OLED_DrawRect(&holed,40,20);
PAL_OLED_SetPen(&holed,OLED_COLOR_TRANSPARENT ,1);
PAL_OLED_SetBrush(&holed,BRUSH_BLACK);
PAL_OLED_SetCursor(&holed,70,20);
PAL_OLED_DrawRect(&holed,40,20);
PAL_OLED_SetPen(&holed,OLED_COLOR_TRANSPARENT ,1);
PAL_OLED_SetBrush(&holed,BRUSH_BLACK);
PAL_OLED_SetCursor(&holed,65,30);
PAL_OLED_DrawCircle(&holed,5);
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}
基本字符的显示
PAL_OLED_Clear(&holed);
PAL_OLED_SetCursor(&holed,5,20);
PAL_OLED_SetPen(&holed,OLED_COLOR_WHITE ,1);
PAL_OLED_SetBrush(&holed,BRUSH_TRANSPARENT);
PAL_OLED_DrawString(&holed,"Hello World");
PAL_OLED_SetCursor(&holed,5,30);
PAL_OLED_Printf(&holed,"Today is %02d/%02d/%02d",23,12,26);
PAL_OLED_SendBuffer(&holed);
文本框功能
改变字体
1.
复制粘贴到front文件夹
调出命令行
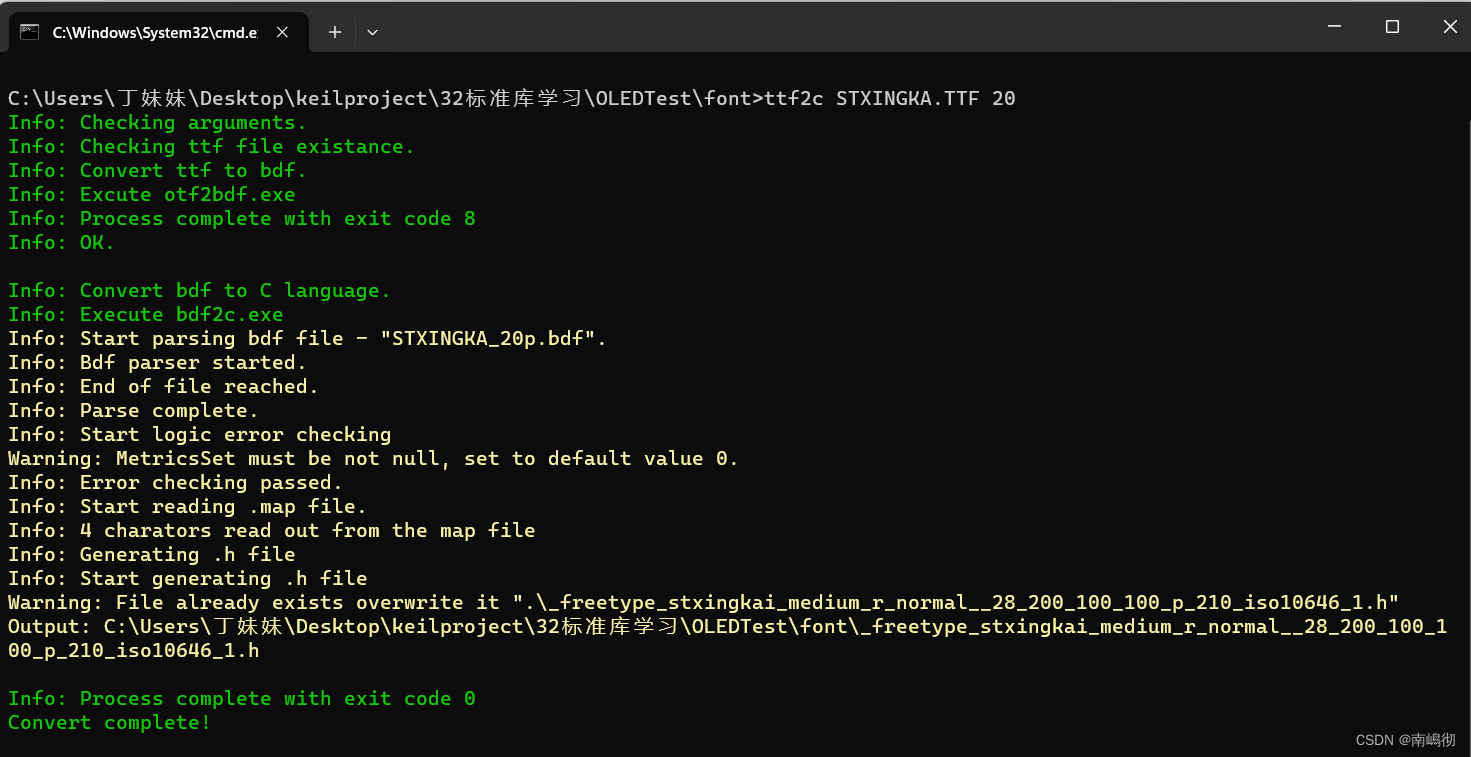
输入字体的文件名 在font后面输入完整的文件名(对应字体的文件名)按回车
生成一个头文件
引用头文件
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
#include "_freetype_stliti_medium_r_normal__28_200_100_100_p_230_iso10646_1.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
PAL_OLED_Clear(&holed);
PAL_OLED_SetFont(&holed,&_freetype_stliti_medium_r_normal__28_200_100_100_p_230_iso10646_1);
PAL_OLED_SetCursor(&holed,(PAL_OLED_GetScreenWidth(&holed) - PAL_OLED_GetStrWidth(&holed,"不吃牛肉")) /2,40);
PAL_OLED_DrawString(&holed,"不吃牛肉");
PAL_OLED_SetFont(&holed,&default_font);
PAL_OLED_SetCursor(&holed,(PAL_OLED_GetScreenWidth(&holed) - PAL_OLED_GetStrWidth(&holed,"Hello World"))/2,60);
PAL_OLED_DrawString(&holed,"Hello World");
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}
进入网站 把图片转化为二进制 就可以输出图片
https://javl.github.io/image2cpp/ (网站地址)
选择图片
声明一个数组用来缓存二进制
#include "stm32f10x.h"
#include "stm32f10x_pal.h"
#include "stm32f10x_pal_i2c.h"
#include "stm32f10x_pal_oled.h"
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
static const uint8_t image[] = {// 'huaqiang', 128x64px
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xfe, 0x7f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf8, 0x1f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xdf, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf5, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xf8, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfd, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xf3, 0xff, 0xff, 0xf8, 0x9f, 0xff, 0xf0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff,
0xe5, 0xff, 0xff, 0xf8, 0x1f, 0x9f, 0xf0, 0x7f, 0xff, 0xff, 0xff, 0xff, 0x87, 0xff, 0xff, 0xff,
0xf1, 0xff, 0xff, 0xfc, 0x1f, 0xff, 0xfe, 0x3f, 0xff, 0xff, 0xff, 0xff, 0x8f, 0xff, 0xff, 0xc0,
0xe0, 0x3f, 0xff, 0xf8, 0x3f, 0xff, 0xf0, 0x3f, 0xff, 0xff, 0xff, 0xff, 0x3d, 0xff, 0xe2, 0x81,
0x08, 0x0f, 0xff, 0xe0, 0x03, 0xff, 0xfd, 0xbf, 0xff, 0xff, 0xff, 0xff, 0xf9, 0xf3, 0xc6, 0xc0,
0x00, 0x0f, 0xff, 0x80, 0x00, 0xff, 0xf8, 0x3f, 0xff, 0xff, 0xff, 0xff, 0xe3, 0xe7, 0x83, 0xc2,
0x00, 0x0f, 0xfe, 0x00, 0x00, 0x3f, 0xf1, 0xdf, 0xff, 0xff, 0xff, 0xff, 0x17, 0xcf, 0xab, 0xf0,
0x00, 0x07, 0x0c, 0x00, 0x10, 0x3f, 0xd0, 0xff, 0xff, 0xff, 0xff, 0xff, 0x27, 0xcf, 0xdf, 0xf7,
0x00, 0x07, 0x04, 0x00, 0x00, 0x1c, 0x20, 0xb7, 0xff, 0xff, 0xff, 0xfe, 0x0f, 0x9f, 0xbf, 0xfe,
0x00, 0x07, 0xc0, 0x00, 0x00, 0x10, 0x00, 0x11, 0xff, 0xff, 0xff, 0xfc, 0x4f, 0x1e, 0x3f, 0xff,
0x00, 0x07, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x11, 0xff, 0xff, 0xff, 0xfc, 0x9f, 0x3a, 0x2f, 0xfb,
0x00, 0x07, 0xf0, 0x00, 0x00, 0x02, 0x00, 0x01, 0xff, 0xff, 0xff, 0xec, 0x1e, 0x78, 0x0f, 0xfd,
0x80, 0x0f, 0xf0, 0x00, 0x00, 0x02, 0x00, 0x07, 0xff, 0xff, 0xff, 0xfd, 0x36, 0x7c, 0x03, 0x7f,
0x84, 0x0f, 0xe0, 0x1f, 0xe0, 0x04, 0x0e, 0x07, 0xff, 0xff, 0xff, 0xfa, 0x3c, 0xf0, 0x03, 0xff,
0x84, 0x0f, 0xec, 0x90, 0x3c, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf2, 0x7c, 0xe4, 0x2b, 0xff,
0x00, 0x07, 0xfc, 0x04, 0x80, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xf4, 0x5c, 0xc4, 0x23, 0xff,
0x00, 0x07, 0xc0, 0xf8, 0x00, 0x71, 0xff, 0xf7, 0xff, 0xff, 0xff, 0xf4, 0xff, 0xac, 0x14, 0x5f,
0x00, 0x07, 0xd0, 0x07, 0xf2, 0x06, 0x00, 0x0f, 0x03, 0x0f, 0xff, 0xac, 0x00, 0x14, 0x45, 0xff,
0xc0, 0x07, 0xff, 0x00, 0x00, 0x00, 0x00, 0x1f, 0x0f, 0xff, 0xff, 0xc8, 0x00, 0x54, 0x0c, 0x00,
0xc0, 0x00, 0xfe, 0x01, 0x00, 0x38, 0x00, 0x1f, 0xff, 0xff, 0xff, 0x70, 0x00, 0x50, 0x00, 0x00,
0x30, 0x03, 0xe0, 0x00, 0x00, 0xf8, 0x00, 0x1f, 0xff, 0xff, 0xfe, 0x7e, 0x00, 0x32, 0x3f, 0x7c,
0x00, 0x02, 0x38, 0x00, 0x00, 0x3e, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x7f, 0x80, 0x03, 0xff, 0xf8,
0x00, 0x07, 0xf8, 0x9f, 0xd0, 0x3e, 0xff, 0xff, 0xff, 0xff, 0xfc, 0x43, 0xf8, 0x03, 0xff, 0xe7,
0x80, 0x07, 0xf0, 0x1f, 0xf2, 0x04, 0xff, 0xf7, 0xff, 0xff, 0xfc, 0x20, 0xff, 0xc3, 0xff, 0x8f,
0x40, 0x07, 0xf0, 0xbf, 0xcc, 0x1c, 0xfd, 0xf1, 0xff, 0xff, 0xfd, 0x40, 0x00, 0xe0, 0xff, 0xff,
0x40, 0x07, 0xf1, 0x0f, 0xc6, 0x1c, 0xff, 0xd1, 0xff, 0xff, 0xfd, 0xa0, 0x40, 0x50, 0x79, 0xff,
0x40, 0x07, 0xf9, 0x3f, 0xe4, 0x1c, 0xe0, 0x17, 0xff, 0xff, 0xff, 0xf8, 0x01, 0xf0, 0x3f, 0xff,
0x40, 0x07, 0xf9, 0x3f, 0xe6, 0x3c, 0x87, 0x07, 0xff, 0xff, 0xff, 0xf0, 0x00, 0x00, 0x0f, 0xff,
0x58, 0x07, 0xfa, 0x90, 0x66, 0x38, 0x0f, 0x83, 0xdf, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x03, 0xff,
0x48, 0x07, 0xfb, 0x90, 0x06, 0x38, 0x07, 0xc3, 0xbf, 0xff, 0xfe, 0x91, 0xc6, 0x00, 0xbd, 0xff,
0x3c, 0x07, 0xfb, 0x94, 0x06, 0x78, 0x07, 0x83, 0x7f, 0xff, 0xfc, 0x00, 0x3f, 0x00, 0x38, 0x7e,
0xbc, 0x07, 0xff, 0xc2, 0x8e, 0x7c, 0x07, 0xc7, 0xff, 0xff, 0xf8, 0x10, 0x03, 0x00, 0x30, 0x43,
0x3c, 0x07, 0xfd, 0xc0, 0x16, 0x3c, 0x00, 0x01, 0xff, 0xff, 0xf8, 0x18, 0x00, 0x25, 0x40, 0x04,
0x7c, 0x07, 0xf8, 0x60, 0x08, 0x3c, 0x00, 0x00, 0x7f, 0xff, 0xfc, 0x18, 0x00, 0x02, 0x10, 0x18,
0x7e, 0x07, 0xfc, 0x3f, 0x80, 0x78, 0x08, 0x0f, 0xff, 0xff, 0xfc, 0x02, 0x00, 0x00, 0x10, 0x7b,
0xfe, 0x1f, 0xfc, 0x00, 0x00, 0x7f, 0x00, 0x1f, 0xff, 0xff, 0xfc, 0x00, 0x40, 0x00, 0x00, 0xfb,
0xfc, 0x0f, 0xfc, 0x00, 0x01, 0xff, 0x80, 0x3f, 0xff, 0xff, 0xfc, 0x00, 0x08, 0x00, 0x00, 0xfb,
0xff, 0xff, 0xf8, 0x00, 0x01, 0xff, 0xc0, 0x7f, 0xff, 0xff, 0xfc, 0x00, 0x01, 0x80, 0x00, 0xfb,
0xff, 0xff, 0xf8, 0x00, 0x02, 0x7f, 0xe4, 0x7f, 0xff, 0xff, 0xfc, 0x00, 0x00, 0x00, 0x00, 0xfb,
0xff, 0xff, 0xf8, 0x00, 0x00, 0x7f, 0xc0, 0x7f, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x00, 0xe0, 0x00,
0xff, 0xff, 0xfc, 0x00, 0x03, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x00, 0x07, 0xe0,
0xff, 0xff, 0xfc, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x00, 0x00, 0x00,
0xff, 0xff, 0xfc, 0x00, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x00, 0x00, 0x00,
0xff, 0xff, 0xfe, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x00, 0x00, 0x00,
0xff, 0xff, 0xff, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x01, 0x00, 0x00,
0xff, 0xff, 0xff, 0x80, 0x0b, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x40, 0x00, 0x01, 0x80, 0x00,
0xff, 0xff, 0xff, 0xe0, 0xfc, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0x60, 0x00, 0x01, 0x00, 0x00,
0xff, 0xff, 0xff, 0xe0, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0xe0, 0x00, 0x01, 0x00, 0x00,
0xff, 0xff, 0xff, 0x00, 0x07, 0xff, 0xff, 0xff, 0xff, 0xfd, 0xfe, 0xc0, 0x00, 0x01, 0x00, 0x00,
0xff, 0xff, 0xc0, 0x00, 0x01, 0xff, 0xff, 0xff, 0xff, 0xff, 0xfe, 0xc0, 0x00, 0x01, 0x80, 0x00,
0xff, 0xff, 0xff, 0x00, 0x0f, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x80, 0x0c, 0x01, 0x80, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x40, 0x0f, 0x81, 0x80, 0x00,
0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0xff, 0x40, 0x0f, 0xe1, 0x80, 0x00};
static PalI2C_HandleTypeDef hi2c1;
static PalOled_HandleTypeDef holed;
int main(void)
{
PAL_Init();
//初始化iic
hi2c1.Init.I2Cx = I2C1;
hi2c1.Init.I2C_ClockSpeed = 400000;
hi2c1.Init.I2C_DutyCycle = I2C_DutyCycle_2;
PAL_I2C_Init(&hi2c1);
//初始化oled屏幕
holed.Init.hi2c = &hi2c1;
holed.Init.SA0 = RESET;
PAL_OLED_Init(&holed);
//生成图片
PAL_OLED_Clear(&holed);
PAL_OLED_SetPen(&holed,PEN_COLOR_WHITE,1);
PAL_OLED_SetBrush(&holed,BRUSH_BLACK);
PAL_OLED_SetCursor(&holed,0,0);
PAL_OLED_DrawBitmap(&holed,128,64,image);
PAL_OLED_SendBuffer(&holed);
while(1)
{
}
}