#include<stdio.h>
#include<stdlib.h>
typedef struct node{
int num;
struct node *next;
}node;
node *create(int n)
{
node* head,*p,*q;
head = (node*)malloc(sizeof(node));
head->num=0;
head->next= NULL;
p=head;
for(int i=0;i<n;i++){
q= (node*)malloc(sizeof(node));
q->num=i;
p->next=q;
p=q;
}
p->next=NULL;
return head;
}
void insert(node* p,int n){
node *charu =(node*)malloc(sizeof(node));
charu->num=n;
charu->next=p->next;
p->next=charu;
}
void printNode(node* head)
{
node *p=head->next;
while (p!= NULL){
printf("node: %d\n",p->num);
p=p->next;
}
}
void del(node* head,int val ){
node* prev =head;
node *p=head->next;
while(p!=NULL){
if(p->num==val){
prev->next=p->next;
free(p);
break;
}
else{
prev=p;
p=p->next;
}
}
}
int main(){
int n;
node* head=create(10);
printf("当前链表的所有节点:\n");
printNode(head);
printf("请输入需要插入的结点编号:\n");
scanf("%d",&n);
insert(head,n);
printf("插入之后的链表的节点:\n");
printNode(head);
printf("请输入需要删除的结点编号:\n");
scanf("%d",&n);
del(head,n);
printf("删除之后的链表的节点:\n");
printNode(head);
return 0;
}
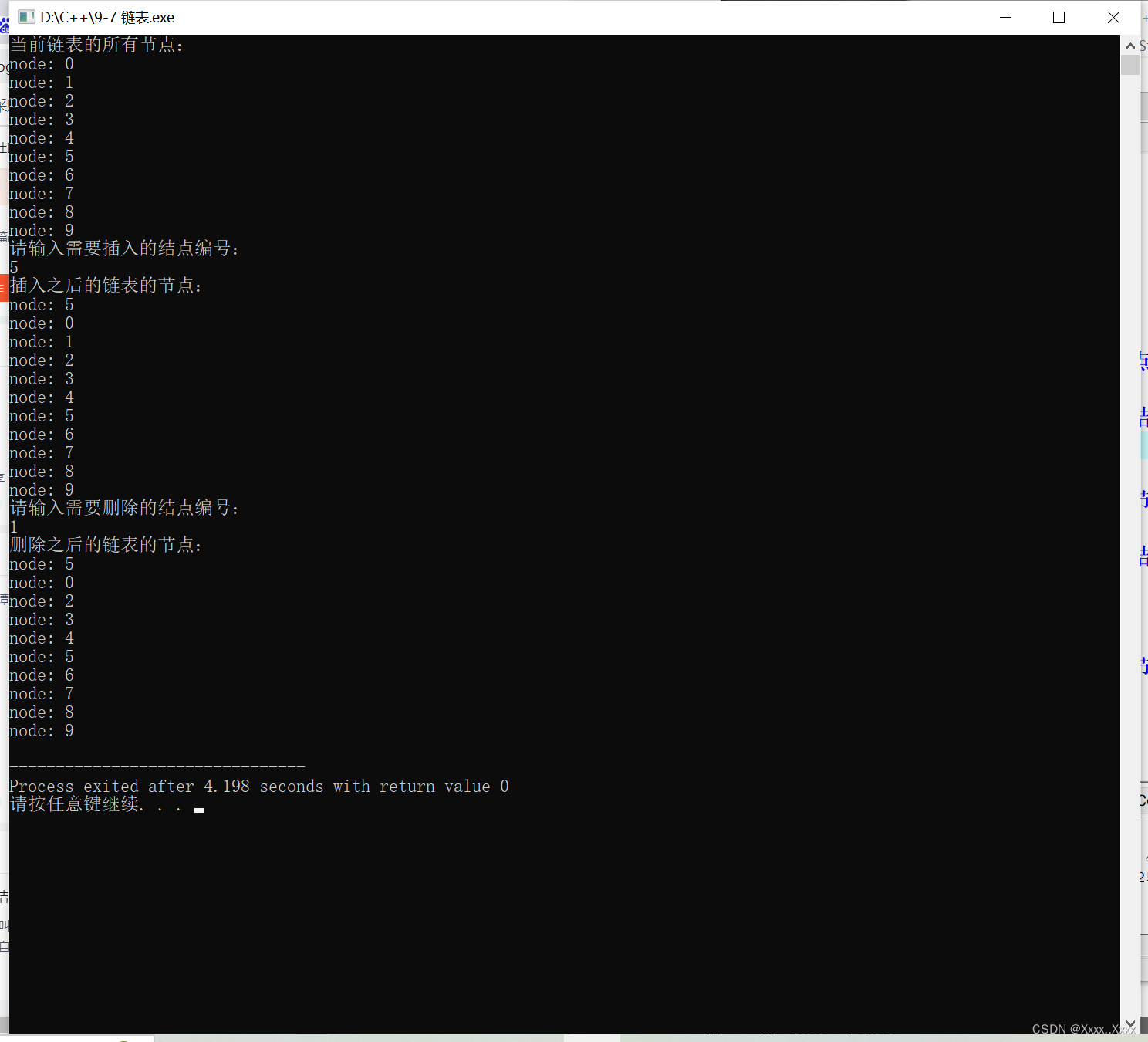