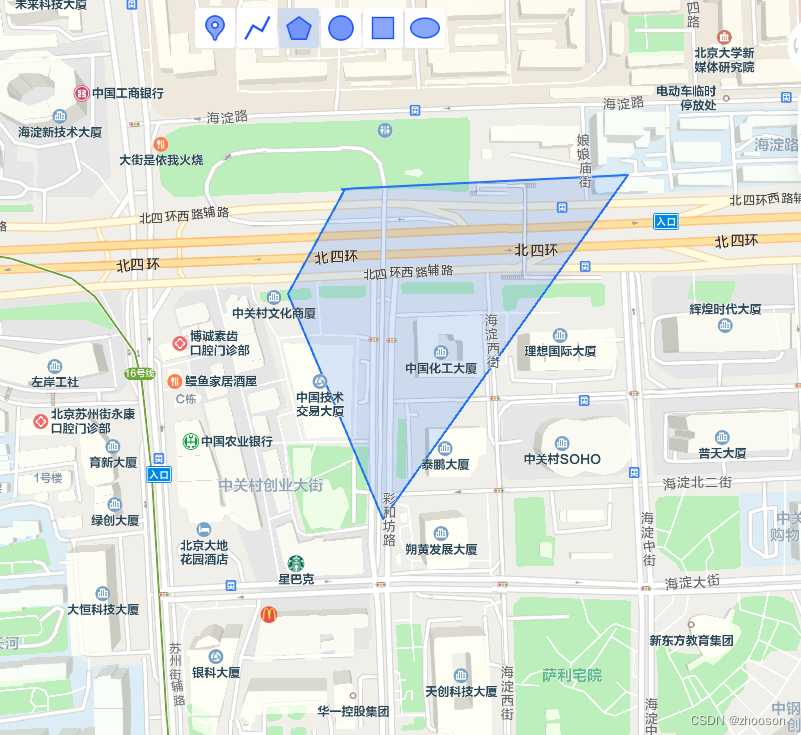
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>地图</title>
</head>
<script src="https://cdn.bootcdn.net/ajax/libs/vue/2.7.4/vue.min.js"></script>
<script
type="text/javascript"
src="https://res.wx.qq.com/open/js/jweixin-1.3.2.js"
></script>
<script
charset="utf-8"
type="text/javascript"
src="https://map.qq.com/api/gljs?libraries=tools,geometry&v=1.exp&key=OXQ-JNFCT"
></script>
<style type="text/css">
html,
body {
height: 100vh;
margin: 0px;
padding: 0px;
}
#my_app {
height: 100%;
}
#container {
width: 100%;
height: 80%;
}
#toolControl {
position: absolute;
top: 10px;
left: 0px;
right: 0px;
margin: auto;
width: 252px;
z-index: 1001;
}
.toolItem {
width: 30px;
height: 30px;
float: left;
margin: 1px;
padding: 4px;
border-radius: 3px;
background-size: 30px 30px;
background-position: 4px 4px;
background-repeat: no-repeat;
box-shadow: 0 1px 2px 0 #e4e7ef;
background-color: #ffffff;
border: 1px solid #ffffff;
}
.toolItem:hover {
border-color: #789cff;
}
.active {
border-color: #d5dff2;
background-color: #d5dff2;
}
#marker {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/marker_editor.png');
}
#polyline {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/polyline.png');
}
#polygon {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/polygon.png');
}
#circle {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/circle.png');
}
#rectangle {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/rectangle.png');
}
#ellipse {
background-image: url('https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/ellipse.png');
}
.next {
margin: 50px auto 0 auto;
width: 300px;
height: 60px;
background: red;
color: #fff;
font-size: 40px;
text-align: center;
}
</style>
<body>
<div id="my_app" v-cloak>
<div id="container"></div>
<div class="next" @click="nextHandler">下一步</div>
<div id="toolControl">
<div
v-for="(item) in list"
:key="item.id"
class="toolItem"
:class="{'active': activeId === item.id}"
:id="item.id"
:title="item.title"
@click="selectItem(item.id)"
></div>
</div>
</div>
</body>
<script>
var one = new Vue({
el: '#my_app',
data: {
activeId: 'polygon', // 初始化默认值
editor: '',
list: [
{
id: 'marker',
title: '点标记',
},
{
id: 'polyline',
title: '折线',
},
{
id: 'polygon',
title: '多边形',
},
{
id: 'circle',
title: '圆形',
},
{
id: 'rectangle',
title: '矩形',
},
{
id: 'ellipse',
title: '椭圆',
},
],
map: null,
locationList: [],
},
mounted() {
this.$nextTick(() => {
this.initMap();
});
},
methods: {
selectItem(id) {
this.activeId = id;
this.editor.setActiveOverlay(id);
},
initMap() {
// 初始化地图
this.map = new TMap.Map('container', {
zoom: 17, // 设置地图缩放级别
center: new TMap.LatLng(39.984104, 116.307503), // 设置地图中心点坐标
});
// 初始化几何图形
var marker = new TMap.MultiMarker({
map: this.map,
});
var polyline = new TMap.MultiPolyline({
map: this.map,
});
var polygon = new TMap.MultiPolygon({
map: this.map,
});
var circle = new TMap.MultiCircle({
map: this.map,
});
var rectangle = new TMap.MultiRectangle({
map: this.map,
});
var ellipse = new TMap.MultiEllipse({
map: this.map,
});
this.editor = new TMap.tools.GeometryEditor({
// TMap.tools.GeometryEditor 文档地址:https://lbs.qq.com/webApi/javascriptGL/glDoc/glDocEditor
map: this.map, // 编辑器绑定的地图对象
overlayList: [
// 可编辑图层 文档地址:https://lbs.qq.com/webApi/javascriptGL/glDoc/glDocEditor#4
{
overlay: marker,
id: 'marker',
},
{
overlay: polyline,
id: 'polyline',
},
{
overlay: polygon,
id: 'polygon',
},
{
overlay: circle,
id: 'circle',
},
{
overlay: rectangle,
id: 'rectangle',
},
{
overlay: ellipse,
id: 'ellipse',
},
],
actionMode: TMap.tools.constants.EDITOR_ACTION.DRAW, // 编辑器的工作模式
activeOverlayId: this.activeId, // 激活图层
snappable: true, // 开启吸附
});
// 监听绘制结束事件,获取绘制几何图形
this.editor.on('draw_complete', (geometry) => {
console.log('所有顶点坐标集合', geometry); // 每种图形返回值不一样,需要特殊处理
this.locationList.push({ ...geometry, type: this.activeId });
// 多边形处理
if (this.activeId === 'polygon') {
this.computeArea(geometry.paths, this.locationList.length - 1);
}
//... 其他类型的形状 面积 自行处理
});
},
// 计算路径围成的多边形的面积
computeArea(path, index) {
const area = TMap.geometry.computeArea(path);
this.locationList[index].area = area;
},
nextHandler() {
wx.miniProgram.getEnv(function (res) {
console.log(res.miniprogram); // true
if (res.miniprogram) {
wx.miniProgram.navigateTo({
url: `/pages/test/test?lcation=${JSON.stringify(
this.locationList
)}`,
});
}
});
},
},
});
</script>
</html>