效果:
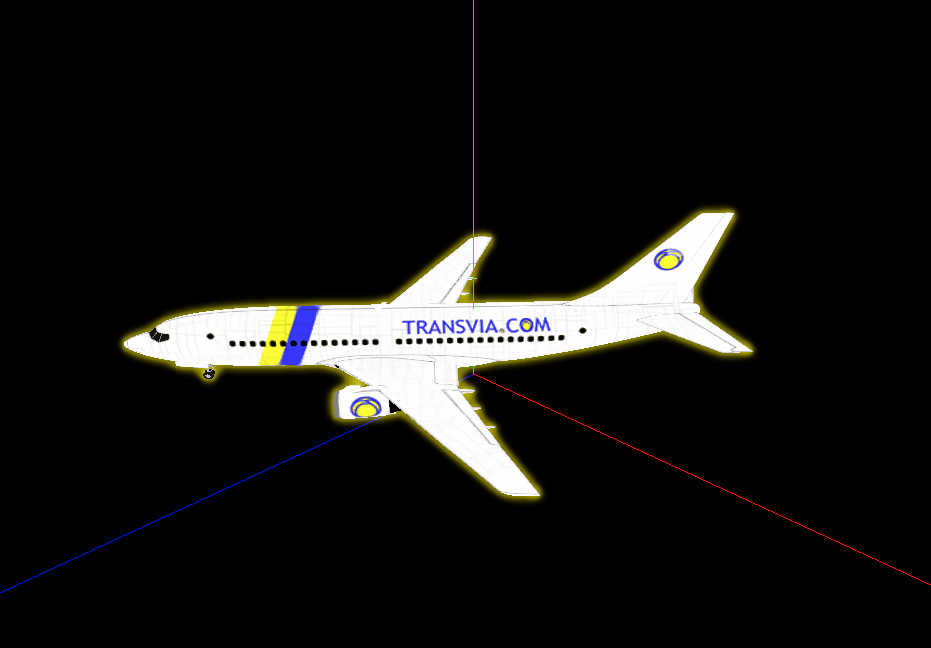
代码 :
<template>
<div>
<el-container>
<el-main>
<div class="box-card-left">
<div id="threejs" style="border: 1px solid red"></div>
<div style="padding: 10px">
<el-button type="primary" plain @click="outline">发光描边</el-button>
</div>
</div>
</el-main>
</el-container>
</div>
</template>
<script>
// 引入轨道控制器扩展库OrbitControls.js
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls.js";
import { GLTFLoader } from "three/examples/jsm/loaders/GLTFLoader.js";
// 效果制作器
import { EffectComposer } from "three/examples/jsm/postprocessing/EffectComposer.js";
// 渲染通道
import { RenderPass } from "three/examples/jsm/postprocessing/RenderPass.js";
// 发光描边OutlinePass
import { OutlinePass } from "three/examples/jsm/postprocessing/OutlinePass.js";
export default {
components: { Drawer },
data() {
return {
name: "",
scene: null, // 场景对象
camera: null, // 相机对象
renderer: null,
geometry: null,
group: null,
material: null,
effectComposer: null,
};
},
created() {},
mounted() {
this.name = this.$route.query.name;
this.init();
},
methods: {
goBack() {
this.$router.go(-1);
},
outline() {
// 创建后处理对象,这里我将该对象称作 效果制作器
this.effectComposer = new EffectComposer(this.renderer);
// 创建渲染器通道
const renderPass = new RenderPass(this.scene, this.camera);
this.effectComposer.addPass(renderPass);
// 创建发光描边对象
const outlinePass = new OutlinePass(new this.$three.Vector2(1000, 800), this.scene, this.camera);
// 设置发光描边颜色
outlinePass.visibleEdgeColor.set(0x999000);
// 设置发光描边厚度
outlinePass.edgeThickness = 5;
// 设置发光描边强度
outlinePass.edgeStrength = 7;
// 设置闪烁频率
outlinePass.pulsePeriod = 2;
const obj = [];
this.scene.traverse(item => {
if(item.isMesh) {
obj.push(item);
}
})
outlinePass.selectedObjects = obj;
this.effectComposer.addPass(outlinePass);
this.renderFun();
},
init() {
// 创建场景对象
this.scene = new this.$three.Scene();
// 创建辅助坐标轴对象
const axesHelper = new this.$three.AxesHelper(150);
this.scene.add(axesHelper);
// 创建环境光对象
const ambientLight = new this.$three.AmbientLight(0xffffff);
this.scene.add(ambientLight);
// 创建相机对象
this.camera = new this.$three.PerspectiveCamera(60, 1, 0.01, 1000);
this.camera.position.set(20, 20, 20);
this.camera.lookAt(0, 0, 0);
// 创建gltf加载器对象
const gltfLoader = new GLTFLoader();
let that = this;
gltfLoader.load("/models/gltf/plane.gltf", (gltf) => {
that.scene.add(gltf.scene);
// 创建渲染器对象
this.renderer = new this.$three.WebGLRenderer();
this.renderer.setSize(1000, 800);
this.renderer.render(this.scene, this.camera);
window.document
.getElementById("threejs")
.appendChild(this.renderer.domElement);
// 创建相机空间轨道控制器
const controls = new OrbitControls(this.camera, this.renderer.domElement);
controls.addEventListener("change", () => {
this.renderer.render(this.scene, this.camera);
})
});
},
renderFun() {
this.effectComposer.render();
window.requestAnimationFrame(this.renderFun);
},
},
};
</script>
<style lang="less" scoped>
.box-card-left {
display: flex;
align-items: flex-start;
flex-direction: row;
width: 100%;
.box-right {
text-align: left;
padding: 10px;
.xyz {
width: 100px;
margin-left: 20px;
}
.box-btn {
margin-left: 20px;
}
}
}
.el-form-item {
margin: 0;
}
</style>