前言
通过freemarker模板生成的word文档实际上不是真正意义上的ms word标准文档,它仍然是xml文件,而docx实际上是一个压缩文件。所以使用以下方法对freemarker模板生成的word进行优化,保证生成的文件是真正意义上的ms word标准文档。
解决方案
- 使用压缩工具打开docx模板,取出document.xml,如下图:
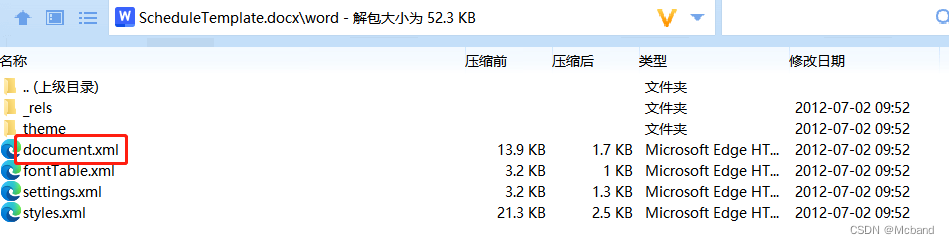
- 将用压缩工具打开后的docx文档里面的document.xml复制出来,并将document.xml后缀改为.ftl,然后进行参数预设。
- 将内容格式化后修改需要替换的内容为freemarker标签,对document.ftl进行参数预设,如下图:
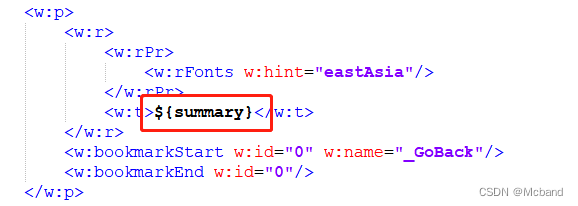
- 文件准备好后存放到某个目录下,docx文件为将要生成好的docx文件样式模版,必须要有的。如下图:

- 程序代码
import freemarker.template.Configuration;
import freemarker.template.Template;
import java.io.*;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Map;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
public class Main {
public static void main(String[] args) {
String templatepath = "E:/test/";
String docxname = "document.docx";
String xmlname = "document.xml";
String tmpxmlpath = "E:/test/temp.xml";
String targetpath = "E:/test/final.docx";
Map<String,Object> data = new HashMap();
data.put("summary","这里是替换的文字");
try {
outputWord(templatepath, docxname, xmlname, tmpxmlpath, targetpath, data);
} catch (Exception e) {
e.printStackTrace();
}
}
private static boolean outputWord(String templatepath, String docxname, String xmlname,
String tmpxmlpath, String targetPath, Map<String, Object> param) throws Exception {
Configuration cfg = new Configuration();
cfg.setDirectoryForTemplateLoading(new File(templatepath));
Template template = cfg.getTemplate(xmlname,"UTF-8");
template.setOutputEncoding("UTF-8");
Writer out = new FileWriter(new File(tmpxmlpath));
template.process(param, out);
if (out != null) {
out.close();
}
File file = new File(tmpxmlpath);
File docxFile = new File(templatepath + "/" + docxname);
ZipFile zipFile = new ZipFile(docxFile);
Enumeration<? extends ZipEntry> zipEntrys = zipFile.entries();
ZipOutputStream zipout = new ZipOutputStream(new FileOutputStream(targetPath));
int len = -1;
byte[] buffer = new byte[1024];
while (zipEntrys.hasMoreElements()) {
ZipEntry next = zipEntrys.nextElement();
InputStream is = zipFile.getInputStream(next);
zipout.putNextEntry(new ZipEntry(next.toString()));
if ("word/document.xml".equals(next.toString())) {
InputStream in = new FileInputStream(file);
while ((len = in.read(buffer)) != -1) {
zipout.write(buffer, 0, len);
}
in.close();
} else {
while ((len = is.read(buffer)) != -1) {
zipout.write(buffer, 0, len);
}
is.close();
}
}
zipout.close();
return true;
}
}
- 会在指定目录下生成文件。如下图:
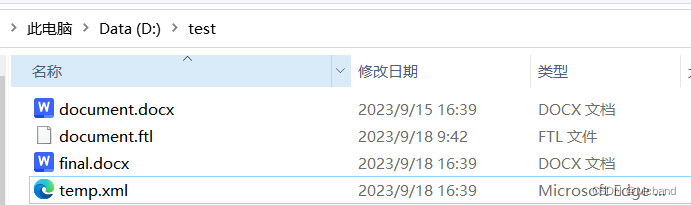