数据结构
数组
特点
- 数组在内存中是连续分配的
- 创建时要指明数组的大小
数组名
代表首地址,索引从0
开始,到数组的长度-1
- 数组一旦创建好,大小不可以改变
- 使用索引
- 获取索引位置的值
arr[index]
- 修改
arr[index] = val
- 删除 (假删除)
- 遍历,将数组中的元素,依次打印出来
使用Java实现更高级的数组
package Arrays;
import java.util.Random;
public class MyArr<T> {
private int capacity = 0;
private int size = 0;
private T[] arr;
public MyArr(int capacity) {
if (capacity < 0) this.capacity = 10;
this.capacity = capacity;
this.arr = (T[]) new Object[capacity];
}
public int getCapacity() {
return capacity;
}
public int getSize() {
return size;
}
public T[] setCapacity(int capacity) {
if (capacity < 0) {
throw new RuntimeException("扩大小异常");
}
this.capacity = capacity;
T[] newNum = (T[]) new Object[capacity];
for (int i = 0; i < this.size; ++i) {
newNum[i] = this.arr[i];
}
return newNum;
}
public void add(T val) {
if (this.size >= this.capacity) {
this.arr = setCapacity(2 * this.capacity);
}
this.arr[this.size++] = val;
}
public boolean removeByIndex(int index) {
if (index < 0 || index > this.capacity) {
throw new RuntimeException("数组越界");
}
for (int i = index; i < size - 1; ++i) {
arr[i] = arr[i + 1];
}
size--;
if (size < this.capacity / 4 && this.capacity > 4) {
arr = setCapacity(this.capacity / 4);
}
return true;
}
public void modify(int index, T val) {
if (index < 0 || index > size - 1) {
throw new RuntimeException("数组越界");
}
arr[index] = val;
}
public int locateVal(T val) {
for (int i = 0; i < size; ++i) {
if (arr[i] == val) {
return i;
}
}
return -1;
}
@Override
public String toString() {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append('[');
for (int i = 0; i < this.size - 1; ++i) {
stringBuffer.append(arr[i] + ",");
}
if(size>0) stringBuffer.append(arr[size - 1]);
stringBuffer.append(']');
return stringBuffer.toString();
}
}
对应习题
class Solution {
public int removeDuplicates(int[] nums) {
int p=0;
int q = 1;
if(nums==null||nums.length==0)return 0;
while(q<nums.length){
if(nums[p]!=nums[q]){
if(q-p>1)nums[p+1]=nums[q];
p++;
}
q++;
}
return p+1;
}
}
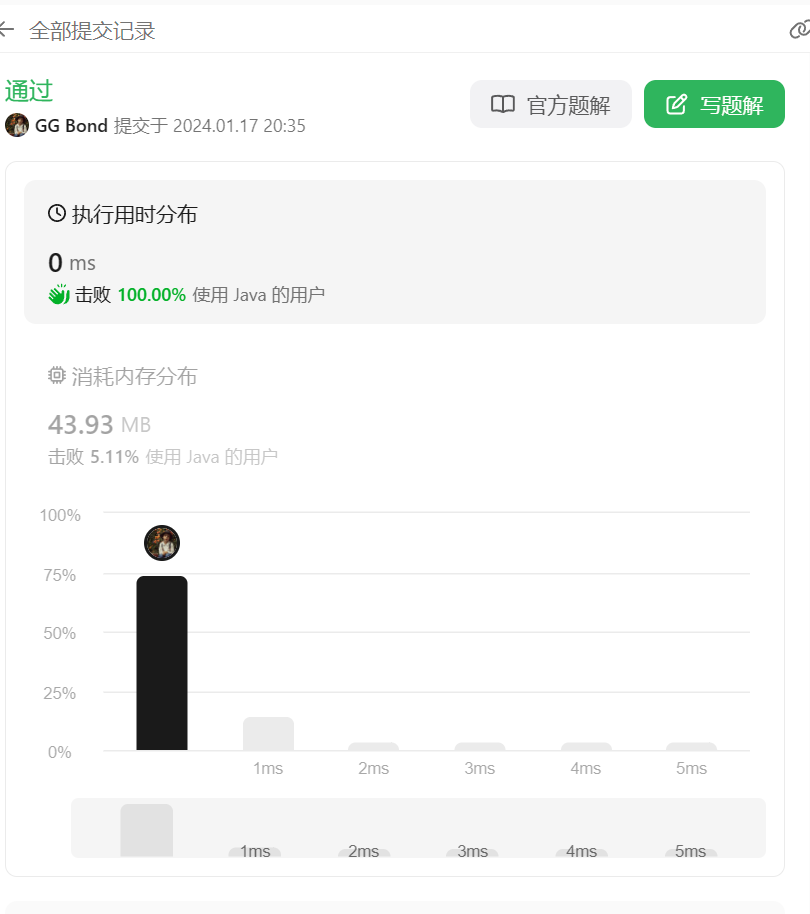
class Solution {
public int[] twoSum(int[] nums, int target) {
int[] numsSum = new int[2];
HashMap<Integer,Integer> hashMap = new HashMap<>();
for(int i=0;i<nums.length;++i){
if(hashMap.containsKey(target - nums[i])){
return new int[]{i, hashMap.get(target - nums[i])};
}
hashMap.put(nums[i],i);
}
return null;
}
}
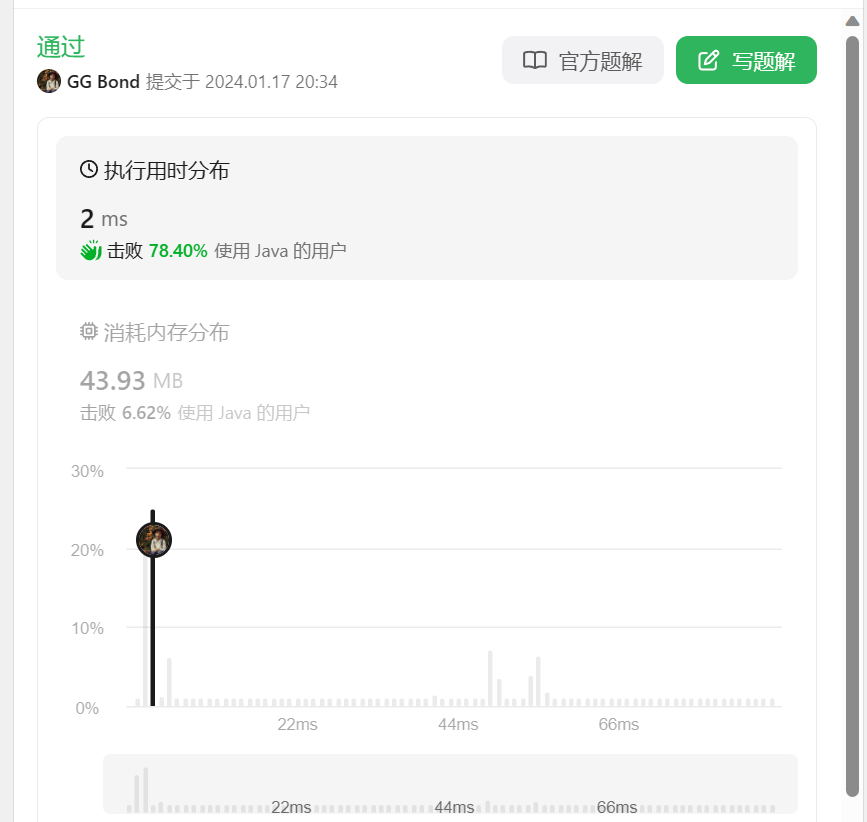
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
lens = len(nums)
dic_num = {}
for i in range(0,lens):
if(target-nums[i]) in dic_num:
return [i,dic_num.get(target-nums[i])]
dic_num[nums[i]] = i
return []