效果图
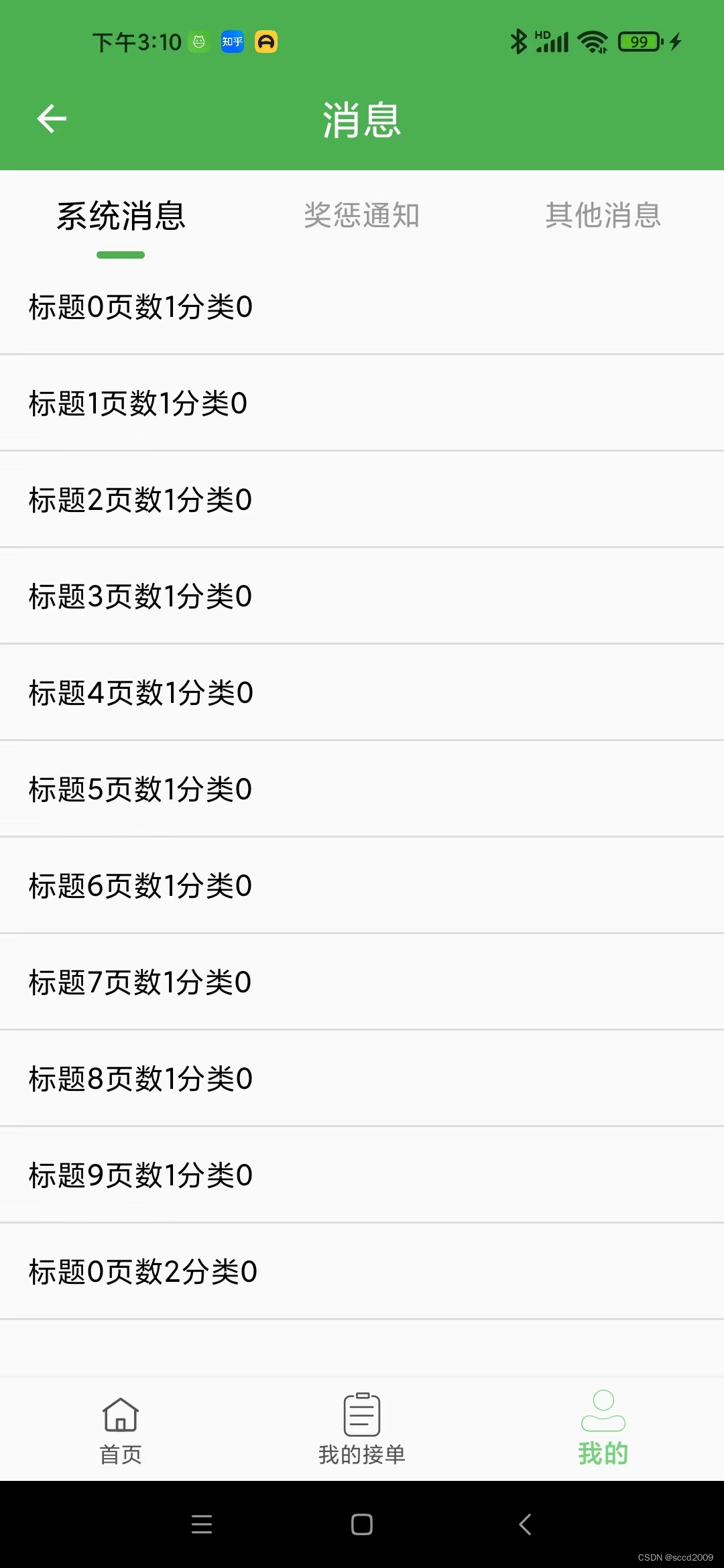
XML?
<androidx.coordinatorlayout.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
android:theme="@style/customTheme">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/teal_900"
app:navigationIcon="@drawable/abc_vector_test"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="消息"
android:textColor="@color/white"
android:textSize="22sp" />
</androidx.appcompat.widget.Toolbar>
<LinearLayout
android:id="@+id/layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingBottom="@dimen/dp_20"
android:orientation="vertical"
android:layout_marginTop="?attr/actionBarSize">
<com.duoduomi.app.widget.HomeTabLayout
android:id="@+id/mTableLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:tabIndicator="@drawable/shape_indicator"
app:tabRippleColor="#00000000"
app:tabMaxWidth="200dp"
app:tabMinWidth="100dp"
app:tabTextColor="#999"
app:tabSelectedTextColor="@color/black"
app:tabIndicatorColor="@color/teal_900"
app:tabTextAppearance="@style/myTabTextAppearance"
/>
<com.scwang.smartrefresh.layout.SmartRefreshLayout
android:id="@+id/smart_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingBottom="@dimen/dp_50"
android:orientation="vertical"
>
<com.scwang.smartrefresh.layout.header.ClassicsHeader
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<com.scwang.smartrefresh.layout.footer.ClassicsFooter
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:srlClassicsSpinnerStyle="Translate" />
</com.scwang.smartrefresh.layout.SmartRefreshLayout>
</LinearLayout>
</androidx.coordinatorlayout.widget.CoordinatorLayout>
Bean模型数据
public class MessageBean {
public Long messageId;
public String title;
public String content;
public String isType;
public Boolean isRead;
public Long memberId;
public void setMessageId(Long messageId){
this.memberId=messageId;
}
public Long getMessageId(){return messageId;}
public void setTitle(String title){
this.title=title;
}
public String getTitle(){return title;}
public void setContent(String content){
this.content=content;
}
public String getContent(){return content;}
public void setIsType(String isType){
this.isType=isType;
}
public String getIsType(){return isType;}
public void setIsRead(Boolean isRead){
this.isRead=isRead;
}
public Boolean getIsRead(){return isRead;}
public void setMemberId(Long memberId){
this.memberId=memberId;
}
public Long getMemberId(){return memberId;}
}
item项布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="15dp">
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="标题"
android:textColor="@android:color/black"
android:textAlignment="textStart"
android:textSize="16sp" />
</LinearLayout>
适配器
public class ItemMessageAdapter extends BaseQuickAdapter<MessageBean, BaseViewHolder> {
private Context mContext;
List<MessageBean> list;
//选中的列表项索引值
public ItemMessageAdapter(int layoutResId, List<MessageBean> list) {
super(layoutResId,list);
this.list=list;
}
@Override
protected void convert(@NonNull BaseViewHolder holder, MessageBean item) {
//holder.setText(R.id.text,item);
holder.setText(R.id.text,item.getTitle());
}
}
Fragement?功能
public class UserMessageFragment extends BaseFragment {
private static final String TAG="UserMessageFragment";
// TODO: Rename parameter arguments, choose names that match
// the fragment initialization parameters, e.g. ARG_ITEM_NUMBER
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
// TODO: Rename and change types of parameters
private String mParam1;
private String mParam2;
private String[] mTitles = {"系统消息", "奖惩通知","其他消息"};
private CommonPopupWindow popupWindow;
public RecyclerView mRecyclerView;
private Integer currentPage=1;
private Boolean hasMoreData=true;
private List<MessageBean> messageBeanList=new ArrayList<>();
private ItemMessageAdapter itemMessageAdapter;
private SmartRefreshLayout mRefreshLayout;
private Integer tabId=0;
private FragmentUserMessageBinding binding;
public UserMessageFragment() {
// Required empty public constructor
}
/**
* Use this factory method to create a new instance of
* this fragment using the provided parameters.
*
* @param param1 Parameter 1.
* @param param2 Parameter 2.
* @return A new instance of fragment UserMessageFragment.
*/
// TODO: Rename and change types and number of parameters
public static UserMessageFragment newInstance(String param1, String param2) {
UserMessageFragment fragment = new UserMessageFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public int getLayoutResID() {
return R.layout.fragment_user_message;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
binding=FragmentUserMessageBinding.inflate(getLayoutInflater());
return binding.getRoot();
}
@Override
public void onActivityCreated(@NonNull Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
ImmersionBar.with(this).statusBarColor(R.color.teal_900).statusBarDarkFont(true).init();
// Set the status bar color to transparent
initFragement();
initTabView();
initLayout();
}
public void initFragement() {
binding.toolbar.setNavigationOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 处理返回事件,通常是回到上一个Fragment
mActivity.onBackPressed();
}
});
//点击详情演示
}
public void initLayout(){
mRecyclerView=binding.recyclerView;
mRefreshLayout=binding.smartLayout;
LinearLayoutManager linearLayoutManager = new LinearLayoutManager(mContext);
linearLayoutManager.setOrientation(RecyclerView.VERTICAL);
mRecyclerView.addItemDecoration(new DividerItemDecoration(getActivity(), DividerItemDecoration.VERTICAL));
mRecyclerView.setLayoutManager(linearLayoutManager);
itemMessageAdapter=new ItemMessageAdapter(R.layout.item_merch_rule,messageBeanList);
getData();
mRecyclerView.setAdapter(itemMessageAdapter);
loadData();
}
public void loadData(){
//下拉刷新
mRefreshLayout.setOnRefreshListener(new OnRefreshListener() {
@Override
public void onRefresh(@NonNull RefreshLayout refreshLayout) {
currentPage = 1;
hasMoreData=true;
mRefreshLayout.setNoMoreData(false);
getData();
itemMessageAdapter.notifyDataSetChanged();
refreshLayout.finishRefresh(500);
}
}).setOnLoadMoreListener(new OnLoadMoreListener() {
@Override
public void onLoadMore(@NonNull RefreshLayout refreshLayout) {
if (hasMoreData) { // 假设 hasMoreData 是你的数据是否还有更多的标志
currentPage++;
getData();
itemMessageAdapter.notifyDataSetChanged();
refreshLayout.finishLoadMore(500);
} else {
refreshLayout.finishLoadMoreWithNoMoreData(); // 当没有更多数据时调用此方法
}
}
});
itemMessageAdapter.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(@NonNull BaseQuickAdapter<?, ?> adapter, @NonNull View view, int position) {
openItem(view,position);
}
});
}
public void getData(){
if(currentPage==1){
//hasMoreData=true;
messageBeanList.clear();
mRefreshLayout.setNoMoreData(false);
}
if(currentPage>5){
//binding.smartLayout.setNoMoreData(true);
hasMoreData=false;
}
//当前页
for(int i=0;i<10;i++){
//list.add("标题"+i+"-"+page);
MessageBean userMessageBean=new MessageBean();
userMessageBean.setMessageId((long) (i+1));
userMessageBean.setTitle("标题"+i+"页数"+currentPage+"分类"+tabId);
userMessageBean.setContent("内容"+i);
messageBeanList.add(userMessageBean);
}
}
private void initTabView() {
List<String> titles = Arrays.asList(mTitles);
HomeTabLayout tabLayout = binding.mTableLayout;
tabLayout.setTitle(titles);
//回调接口
tabLayout.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener(){
@Override
public void onTabSelected(TabLayout.Tab tab) {
//选中事件
Log.d("点击状态","status"+titles.get(tab.getPosition())+tab.getPosition());
tabId=tab.getPosition();
getData();
itemMessageAdapter.notifyDataSetChanged();
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
//未选中事件
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
});
}
public void openItem(View v,Integer position){
popupWindow=new CommonPopupWindow.Builder(getContext())
.setView(R.layout.popup_rule_detail)
.setWidthAndHeight(WindowManager.LayoutParams.MATCH_PARENT, WindowManager.LayoutParams.MATCH_PARENT)
.setBackGroundLevel(0.6f)
.setAnimationStyle(R.anim.photo_dialog_in_anim)
.setViewOnclickListener(new CommonPopupWindow.ViewInterface() {
@Override
public void getChildView(View views, int layoutResId) {
Log.d(TAG,"点击转主账号弹框");
//处理界面事件
ImageView cancel=views.findViewById(R.id.delete);
cancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
popupWindow.dismiss();
}
});
HtmlTextView htmlTextView=views.findViewById(R.id.html_text);
htmlTextView.setHtml(messageBeanList.get(position).getContent());
}
})
.setOutsideTouchable(true)
.create();
popupWindow.showAtLocation(v, Gravity.BOTTOM, 0, 0);
}
}