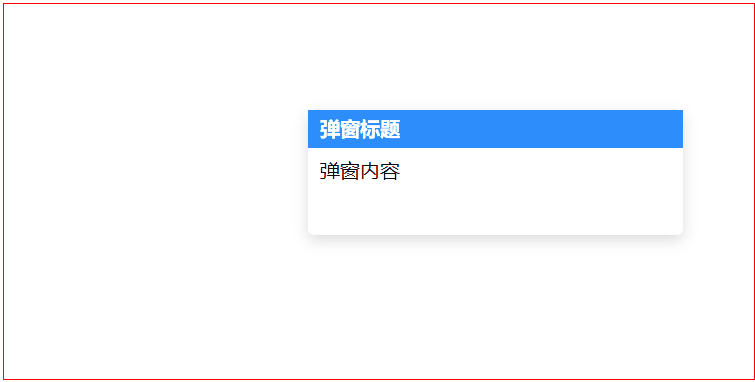
<template>
<div class="container" ref="container">
<div class="drag-box" v-drag>
<div class="win_head">弹窗标题</div>
<div class="win_content">弹窗内容</div>
</div>
</div>
</template>
<script>
export default {
directives: {
drag: {
bind: function (el, binding, vnode) {
let that = vnode.context;
let drag_dom = el;
let drag_handle = el.querySelector(".win_head");
drag_handle.onmousedown = (e) => {
let disX = e.clientX - drag_dom.offsetLeft;
let disY = e.clientY - drag_dom.offsetTop;
let container_dom = that.$refs.container;
document.onmousemove = (e) => {
let left = e.clientX - disX;
let top = e.clientY - disY;
let leftMax =
container_dom.offsetLeft +
(container_dom.clientWidth - drag_dom.clientWidth);
let leftMin = container_dom.offsetLeft + 1;
if (left > leftMax) {
drag_dom.style.left = leftMax + "px";
} else if (left < leftMin) {
drag_dom.style.left = leftMin + "px";
} else {
drag_dom.style.left = left + "px";
}
let topMax =
container_dom.offsetTop +
(container_dom.clientHeight - drag_dom.clientHeight);
let topMin = container_dom.offsetTop + 1;
if (top > topMax) {
drag_dom.style.top = topMax + "px";
} else if (top < topMin) {
drag_dom.style.top = leftMin + "px";
} else {
drag_dom.style.top = top + "px";
}
};
document.onmouseup = () => {
document.onmousemove = null;
document.onmouseup = null;
};
};
},
},
},
};
</script>
<style lang="scss" scoped>
.drag-box {
position: absolute;
top: 100px;
left: 100px;
width: 300px;
height: 100px;
background: #fff;
border-radius: 5px;
box-shadow: 0 4px 12px rgba(0, 0, 0, 0.15);
// 禁止文字被选中
user-select: none;
}
.container {
border: 1px solid red;
width: 600px;
height: 300px;
margin: 30px;
}
.win_head {
background-color: rgb(45, 141, 250);
color: white;
height: 30px;
line-height: 30px;
font-weight: bold;
padding-left: 10px;
cursor: move;
}
.win_content {
padding: 10px;
}
</style>