完成图片拷贝,要求一个线程拷贝- -半,另一个线程拷贝另一 半。
a.找临界资源,找临界区,对临界区上锁解锁即可
#include <func.h>
#include <stdio.h>
pthread_mutex_t mutex;
//拷贝前半部分
void * cphead(void * arg)
{
//打开图片
int ph = open("./1.png", O_RDONLY);
//打开拷贝文件
FILE * pc = fopen("./11.png", "w");
//拷贝前半部分
off_t size = lseek(ph,0,SEEK_END);//文大小件;
ssize_t ter=0;
char a;
int i = 0;
lseek(ph,0,SEEK_SET);
fseek(pc, 0,SEEK_SET);
while(i < size/2)
{ //------临界--------
//上锁------------------------
pthread_mutex_lock(&mutex);
i++;
ter = read(ph,&a,sizeof(a));
fprintf(pc,"%c", a);
lseek(ph,0, SEEK_CUR);
//------临界--------
//解锁-------------------------
pthread_mutex_unlock(&mutex);
}
printf("前半部分拷贝成功\n");
//关闭文件
close(ph);
fclose(pc);
//结束子线程
pthread_exit(NULL);
}
//拷贝后半部分
void * cpend(void * arg)
{
//打开图片
int ph = open("./1.png", O_RDONLY);
//打开拷贝文件
FILE * pc = fopen("./11.png", "w");
//拷贝后半部分
off_t size = lseek(ph,0,SEEK_END);//文大小件;
ssize_t ter=0;
char a;
int i = 0;
lseek(ph, size/2,SEEK_SET);
fseek(pc, size/2,SEEK_SET);
while(1)
{ //------临界--------
//上锁------------------------
pthread_mutex_lock(&mutex);
i++;
ter = read(ph,&a,sizeof(a));
if(ter == 0)
{
break;
}
fprintf(pc,"%c", a);
lseek(ph,0, SEEK_CUR);
//------临界--------
//解锁-------------------------
pthread_mutex_unlock(&mutex);
}
printf("后半部分拷贝成功\n");
//关闭文件
close(ph);
fclose(pc);
//结束子线程
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
//创建互斥锁
pthread_mutex_init(&mutex, NULL);
//创建两个线程
pthread_t tid;
pthread_t tid1;
pthread_create(&tid, NULL, cphead, NULL); //复制前半部分;
pthread_create(&tid1, NULL, cpend, NULL);//复制后半部分;
void* pid;
pthread_join(tid, &pid);//阻塞拷贝1;
pthread_join(tid1,&pid);//阻塞拷贝1;
//销毁锁
pthread_mutex_destroy(&mutex);
return 0;
}

创建两个线程,要求一个线程从文件中读取数据, 另-个线程将读取到的数据打印到终端,类似cat- 个文件。文件cat完
毕后,要结束进程
a.读到一次数据,打印一次数据
#include <func.h>
#include <stdio.h>
#include <semaphore.h>
FILE * ph = NULL;
//信号灯
sem_t sem1, sem2;
char c[128];
void * callbake1(void *arg)
{
//读取内容
int ter = 0;
while(1)
{
/************临界条件*********/
//p操作 -1
if(sem_wait(&sem1) < 0)
{
perror("sem_wait");
return NULL;
}
ter = fscanf(ph,"%s", c);
printf("%d", ter);
/************临界条件*********/
if(sem_post(&sem1) < 0)
{
perror("sem_post");
return NULL;
}
if(ter == EOF)
{
break;
}
}
pthread_exit(NULL);
}
void * callbake2(void * arg)
{
int ter;
//打印到终端
while(1)
{
/************临界条件*********/
//p操作 -1
if(sem_wait(&sem2) < 0)
{
perror("sem_wait");
return NULL;
}
ter = fprintf(stdout, "%s", c);
if(ter = EOF)
{
break;
}
/************临界条件*********/
if(sem_post(&sem2) < 0)
{
perror("sem_post");
return NULL;
}
pthread_exit(NULL);
}
}
int main(int argc, const char *argv[])
{
ph = fopen("./03_lianxi.c", "r");
//创造信号灯;
if(sem_init(&sem1, 0, 1) < 0)
{
perror("sem_init");
return -1;
} //创造信号灯;
if(sem_init(&sem2, 0, 0) < 0)
{
perror("sem_init");
return -1;
}
printf("__%d__", __LINE__);
//创造线程
pthread_t tid1, tid2;
if(pthread_create(&tid1, NULL,callbake1, NULL) < 0)
{
perror("pthread_create");
return -1;
}
if(pthread_create(&tid2, NULL,callbake2, NULL) < 0)
{
perror("pthread_create");
return -1;
}
//阻塞
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
//销毁信号量
sem_destroy(&sem1);
sem_destroy(&sem2);
return 0;
}
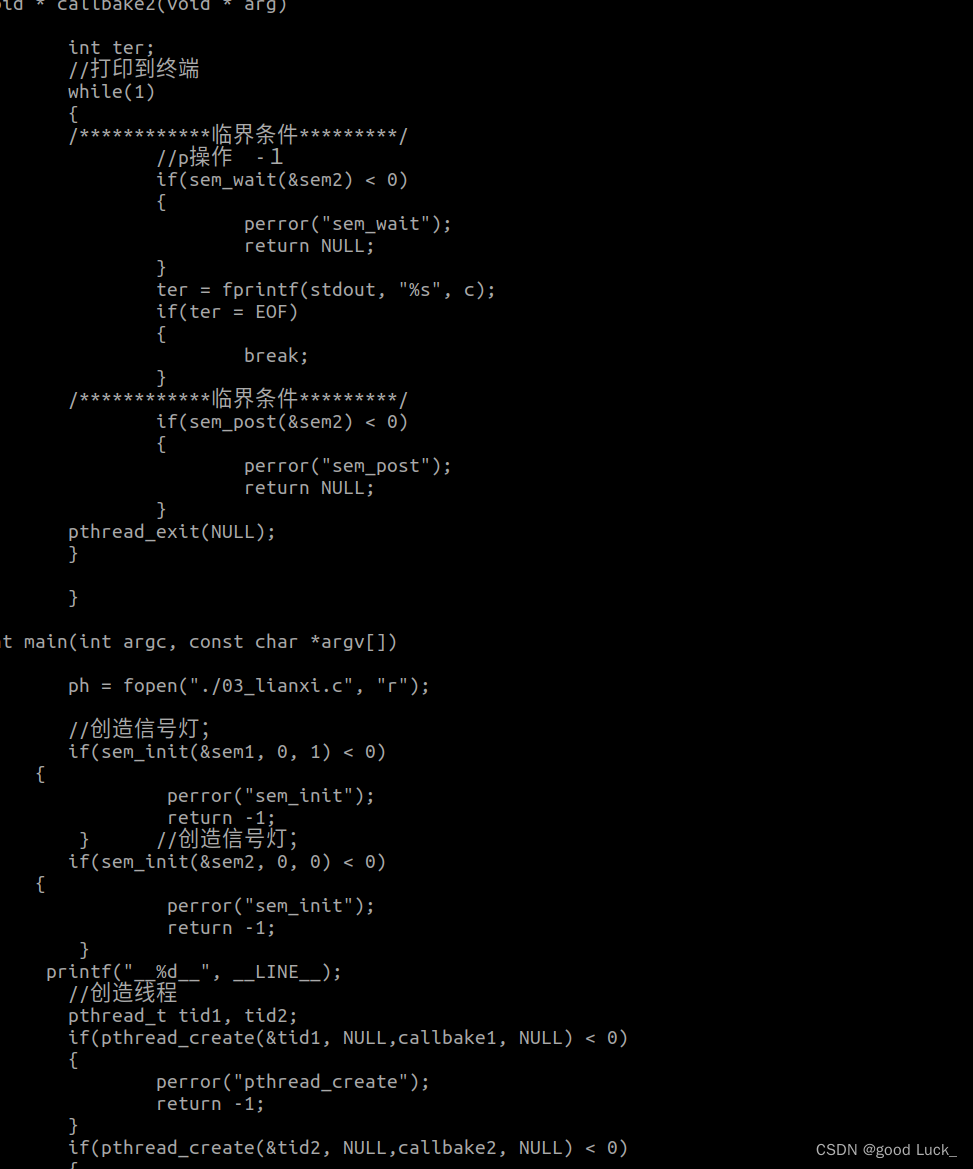