?效果:
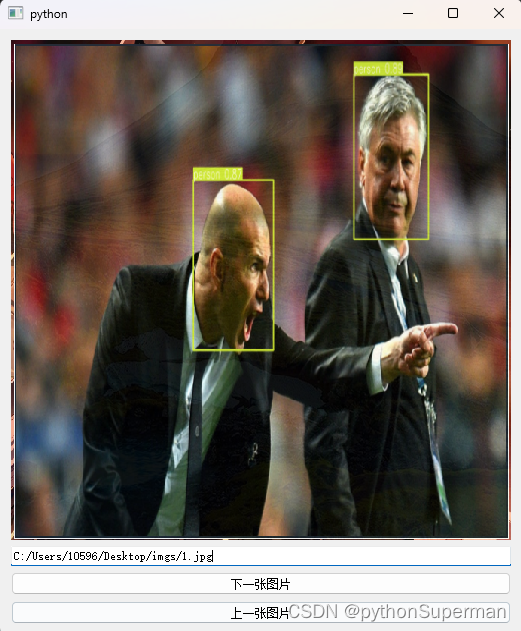
代码?
from PyQt5 import QtWidgets, QtGui
class ImageViewer(QtWidgets.QWidget):
def __init__(self):
super().__init__()
self.selected_imgsPath = []
self.current_image_index = 0
self.label_3 = QtWidgets.QLabel(self)
self.label_3.setFixedSize(500, 500) # 设置标签大小为500x500
self.lineEdit_3 = QtWidgets.QLineEdit(self)
self.next_image_button = QtWidgets.QPushButton("下一张图片", self)
self.next_image_button.clicked.connect(self.showNextImage)
self.prev_image_button = QtWidgets.QPushButton("上一张图片", self)
self.prev_image_button.clicked.connect(self.showPrevImage)
self.layout = QtWidgets.QVBoxLayout(self)
self.layout.addWidget(self.label_3)
self.layout.addWidget(self.lineEdit_3)
self.layout.addWidget(self.next_image_button)
self.layout.addWidget(self.prev_image_button)
self.openImage() # 调用 openImage 函数显示第一张图片
def openImage(self):
self.selected_imgsPath, _ = QtWidgets.QFileDialog.getOpenFileNames(
self, "打开图片", "./pending_images", "*.jpg;;*.png;;All Files(*)")
if len(self.selected_imgsPath) == 0:
self.empty_information()
print('empty!')
return
img = QtGui.QPixmap(self.selected_imgsPath[0]).scaled(500, 500) # 调整图片大小为500x500
self.label_3.setPixmap(img)
self.lineEdit_3.setText(self.selected_imgsPath[0])
self.current_image_index = 0
def showNextImage(self):
if len(self.selected_imgsPath) == 0:
return
self.current_image_index += 1
if self.current_image_index >= len(self.selected_imgsPath):
self.current_image_index = 0
img_path = self.selected_imgsPath[self.current_image_index]
img = QtGui.QPixmap(img_path).scaled(500, 500) # 调整图片大小为500x500
self.label_3.setPixmap(img)
self.lineEdit_3.setText(img_path)
def showPrevImage(self):
if len(self.selected_imgsPath) == 0:
return
self.current_image_index -= 1
if self.current_image_index < 0:
self.current_image_index = len(self.selected_imgsPath) - 1
img_path = self.selected_imgsPath[self.current_image_index]
img = QtGui.QPixmap(img_path).scaled(500, 500) # 调整图片大小为500x500
self.label_3.setPixmap(img)
self.lineEdit_3.setText(img_path)
if __name__ == "__main__":
import sys
app = QtWidgets.QApplication(sys.argv)
viewer = ImageViewer()
viewer.show()
sys.exit(app.exec_())