一、文件下载到本地
import requests
if __name__ == '__main__':
response = requests.get("https://dfcv-shop.oss-cn-hangzhou.aliyuncs.com/dfcv-shop0177bf6b34ee46c68be412d04654439c.jpg")
local_filename = "./dfcv-shop0177bf6b34ee46c68be412d04654439c.jpg"
if response.status_code == 200:
with open(local_filename, 'wb') as file:
file.write(response.content)
print(f"文件 '{local_filename}' 下载成功!")
else:
print("下载失败")
二、服务端对外提供下载资源
from flask import Flask, send_file
app = Flask(__name__)
@app.route('/download')
def download_file():
file_path = 'E:\code\ZhkCode\Dockerfile'
return send_file(file_path, as_attachment=True)
if __name__ == '__main__':
app.run(debug=True)
三、上传资源
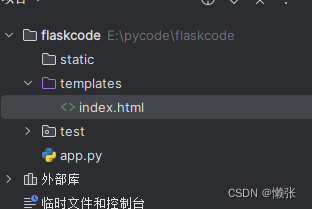
<!DOCTYPE html>
<html>
<head>
<title>文件上传示例</title>
</head>
<body>
<h2>上传文件</h2>
<form method="POST" action="/upload" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
</body>
</html>
from flask import Flask, render_template, request
app = Flask(__name__)
ALLOWED_EXTENSIONS = {'txt', 'pdf', 'png', 'jpg', 'jpeg', 'gif'}
def allowed_file(filename):
return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
@app.route('/')
def upload_form():
return render_template('index.html')
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return 'No file part'
file = request.files['file']
if file.filename == '':
return 'No selected file'
if file and allowed_file(file.filename):
file.save('F:/uploads/' + file.filename)
return 'File uploaded successfully'
else:
return 'Invalid file extension'
if __name__ == '__main__':
app.run(debug=True)