CodePen
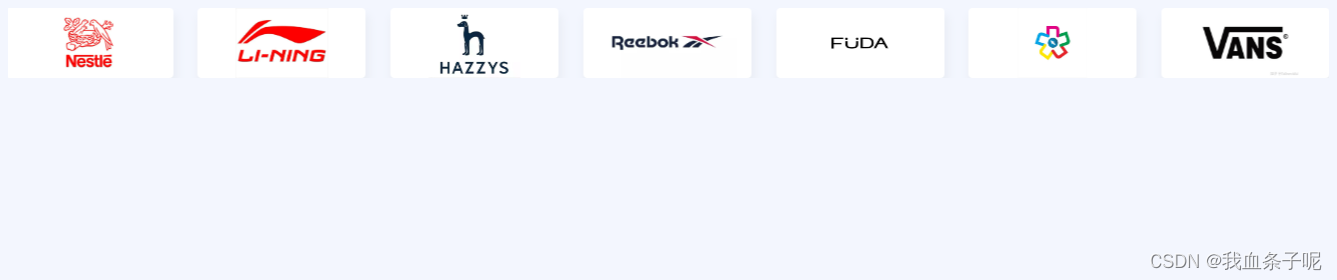
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title></title>
<style>
html,
body {
background: #f3f6fe;
}
.parent {
width: 100%;
height: 70px;
white-space: nowrap;
overflow: hidden;
}
.container-wrapper {
position: relative;
}
.container {
display: inline-block;
white-space: nowrap;
animation: scrollAnimation 20s linear infinite;
}
@keyframes scrollAnimation {
0% {
transform: translateX(0);
}
100% {
transform: translateX(-100%);
}
}
.child {
display: inline-block;
width: 168px;
height: 70px;
background: #fff;
border-radius: 4px;
box-shadow: 2px 8px 8px 2px #eaeef5;
margin-right: 20px;
overflow: hidden;
}
.child > img {
width: 100%;
height: 100%;
object-fit: contain;
}
</style>
</head>
<body>
<div class="parent">
<div class="container-wrapper">
<div class="container" id="container">
<div class="child">
<img src="https://img1.baidu.com/it/u=4192660698,4228364625&fm=253&fmt=auto&app=138&f=JPEG?w=750&h=375" />
</div>
<div class="child">
<img src="https://img0.baidu.com/it/u=2073014214,797944792&fm=253&fmt=auto&app=138&f=JPEG?w=889&h=500" />
</div>
<div class="child">
<img src="https://img1.baidu.com/it/u=1112274159,2885021372&fm=253&fmt=auto&app=138&f=JPG?w=500&h=500" />
</div>
<div class="child">
<img src="https://pic3.zhimg.com/v2-87d99f0c412221d15420a69e2150f78e_b.jpg" />
</div>
<div class="child">
<img src="https://img2.baidu.com/it/u=4206423873,2794900790&fm=253&fmt=auto&app=138&f=JPEG?w=707&h=500" />
</div>
<div class="child">
<img src="https://img0.baidu.com/it/u=3443668923,2767778850&fm=253&fmt=auto&app=138&f=JPEG?w=500&h=375" />
</div>
<div class="child">
<img src="https://img0.baidu.com/it/u=1435678765,4223566504&fm=253&fmt=auto&app=138&f=JPEG?w=527&h=395" />
</div>
<div class="child">
<img src="https://img2.baidu.com/it/u=2883718771,2133249831&fm=253&fmt=auto&app=138&f=JPEG?w=660&h=500" />
</div>
</div>
<div class="container" id="clone-container"></div>
</div>
</div>
<script>
document.addEventListener("DOMContentLoaded", function () {
var container = document.getElementById("container");
var cloneContainer = document.getElementById("clone-container");
container.innerHTML += container.innerHTML;
container.addEventListener("animationiteration", function () {
container.style.transform = "translateX(0)";
});
container.addEventListener("animationend", function () {
container.style.transform = "translateX(0)";
});
setInterval(function () {
cloneContainer.innerHTML = container.innerHTML;
}, 5000);
});
</script>
</body>
</html>