JavaAwtSwing用 GridBagLayout GridBagConstraints 居中
GridBag单行单列居中JLabel
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GridBag单行单列居中JLabel {
static JFrame frame = new JFrame(Thread.currentThread().getStackTrace()[1].getClassName());
static GridBagLayout frameGbl = new GridBagLayout();
static GridBagConstraints frameGbc = new GridBagConstraints();
static {
frame.addWindowListener(new WindowAdapter() {
@Override public void windowClosing(WindowEvent ev) {
System.exit(0);
}
});
frame.setBounds(100, 100, 1024, 768);
frame.setLayout(frameGbl);
}
static JLabel jlb = new JLabel("""
<html>
<style>
#div001{ padding:50px 25px; }
.c1{font-size:16px; color:blue;}
</style>
<div id="div001" style="background:red; ">
<center class=c1 >JLabel</center>
<center class=c1 >内部文字Html区域居中</center>
<center class=c1 >setHorizontalAlignment(JLabel.CENTER);</center>
<center class=c1 >setVerticalAlignment(JLabel.CENTER);</center>
</div>
</html>
""");
static {
jlb.setOpaque(true);
jlb.setBackground(Color.DARK_GRAY);
jlb.setHorizontalAlignment(JLabel.CENTER);
jlb.setVerticalAlignment(JLabel.CENTER);
GridBagLayout gbl = frameGbl; GridBagConstraints gbc = frameGbc;
gbl.columnWidths = new int[] {600};
gbl.rowHeights = new int[] {400};
gbc.fill = GridBagConstraints.BOTH;
frame.add(jlb, gbc);
}
public static void main(String...arguments)throws Exception{
frame.setVisible(true);
}
}
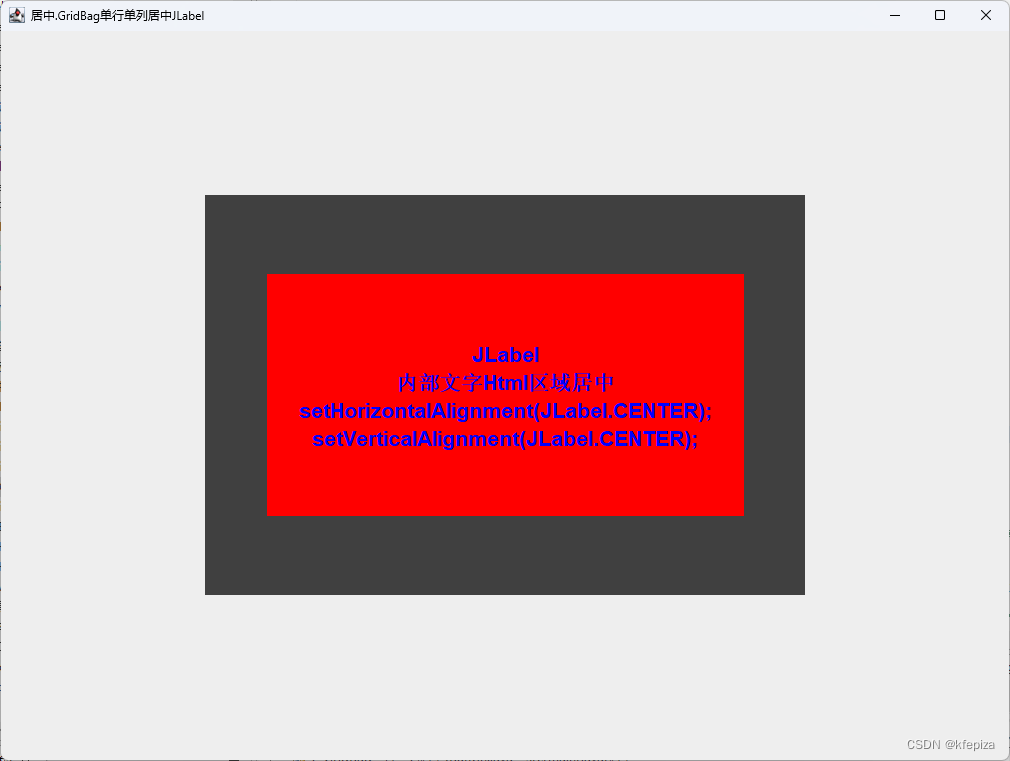
GridBag三行三列居中JLabel
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GridBag三行三列居中JLabel {
static JFrame frame = new JFrame(Thread.currentThread().getStackTrace()[1].getClassName());
static GridBagLayout frameGbl = new GridBagLayout();
static GridBagConstraints frameGbc = new GridBagConstraints();
static {
frame.addWindowListener(new WindowAdapter() {
@Override public void windowClosing(WindowEvent ev) {
System.exit(0);
}
});
frame.setBounds(100, 100, 1024, 768);
frame.setLayout(frameGbl);
}
static JLabel jlb = new JLabel("""
<html>
<style>
#div001{ padding:50px 25px; }
.c1{font-size:16px; color:blue;}
</style>
<div id="div001" style="background:red; ">
<center class=c1 >JLabel</center>
<center class=c1 >内部文字Html区域居中</center>
<center class=c1 >setHorizontalAlignment(JLabel.CENTER);</center>
<center class=c1 >setVerticalAlignment(JLabel.CENTER);</center>
</div>
</html>
""");
static {
jlb.setOpaque(true);
jlb.setBackground(Color.DARK_GRAY);
jlb.setHorizontalAlignment(JLabel.CENTER);
jlb.setVerticalAlignment(JLabel.CENTER);
GridBagLayout gbl = frameGbl; GridBagConstraints gbc = frameGbc;
gbl.columnWidths = new int[] {9999,600,9999};
gbl.rowHeights = new int[] {666,400,666};
gbc.fill = GridBagConstraints.BOTH;
gbc.gridx=1; gbc.gridy=1;
gbc.anchor = GridBagConstraints.WEST;
frame.add(jlb, gbc);
}
public static void main(String...arguments)throws Exception{
frame.setVisible(true);
}
}
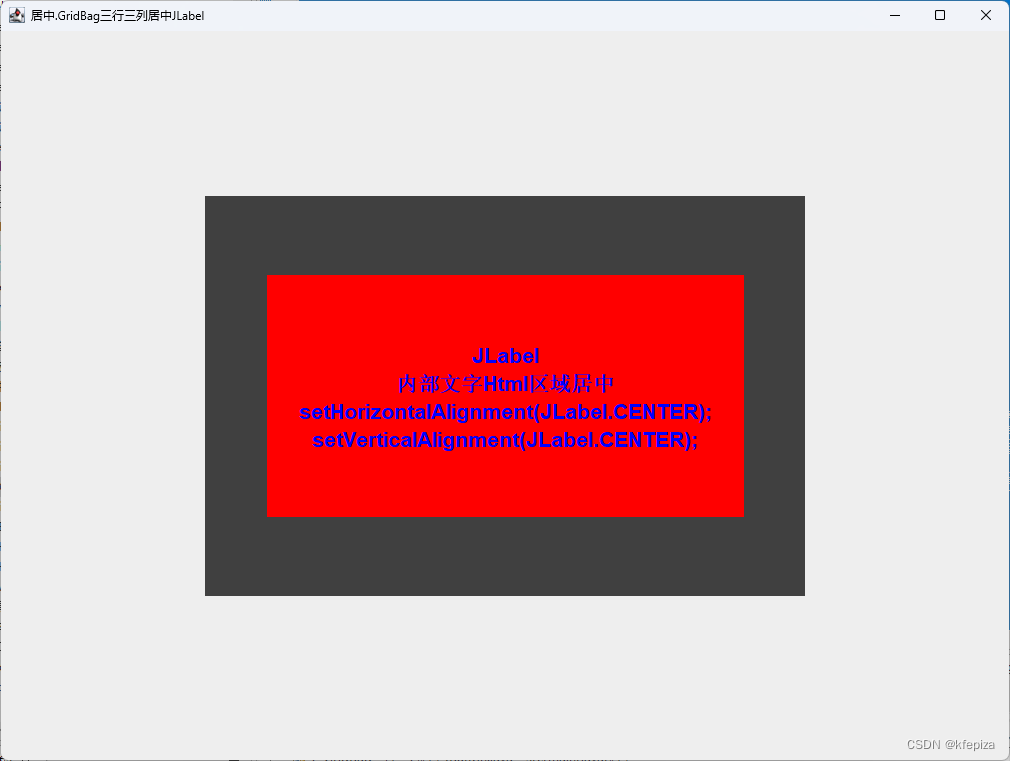
GridBag单行单列居中JButton
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GridBag单行单列居中JButton {
static JFrame frame = new JFrame(Thread.currentThread().getStackTrace()[1].getClassName());
static GridBagLayout frameGbl = new GridBagLayout();
static GridBagConstraints frameGbc = new GridBagConstraints();
static {
frame.addWindowListener(new WindowAdapter() {
@Override public void windowClosing(WindowEvent ev) {
System.exit(0);
}
});
frame.setBounds(100, 100, 800, 600);
frame.setLayout(frameGbl);
}
static JButton jbt = new JButton("jbt001");
static {
GridBagLayout gbl = frameGbl; GridBagConstraints gbc = frameGbc;
gbl.columnWidths = new int[] {300};
gbl.rowHeights = new int[] {100};
gbc.fill = GridBagConstraints.BOTH;
frame.add(jbt, gbc);
}
public static void main(String...arguments)throws Exception{
frame.setVisible(true);
}
}
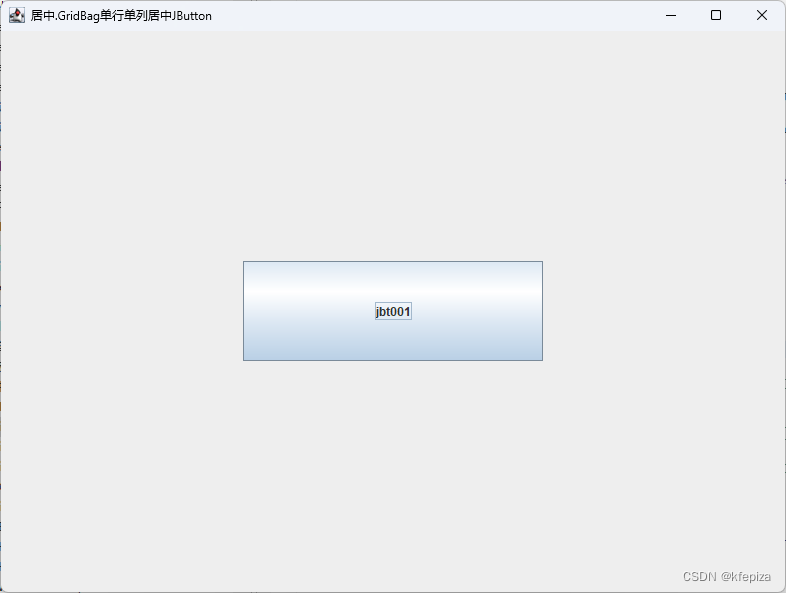
GridBag三行三列居中JButton
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GridBag三行三列居中JButton {
static JFrame frame = new JFrame(Thread.currentThread().getStackTrace()[1].getClassName());
static GridBagLayout frameGbl = new GridBagLayout();
static GridBagConstraints frameGbc = new GridBagConstraints();
static {
frame.addWindowListener(new WindowAdapter() {
@Override public void windowClosing(WindowEvent ev) {
System.exit(0);
}
});
frame.setBounds(100, 100, 1024, 768);
frame.setLayout(frameGbl);
}
static JButton jbt = new JButton("""
<html>
<style>
#div001 center{font-size:20px; color:blue; }
</style>
<div id="div001" style="background:yellow; padding:30px; ">
<center style="font-size:20px; color:black;" >用</center>
<center>GridBagLayout</center>
<center>GridBagConstraints</center>
<center style="font-size:40px; color:black;" >居中</center>
<center >黄色的是HTML区域</center>
</div>
</html>
""");
static {
GridBagLayout gbl = frameGbl; GridBagConstraints gbc = frameGbc;
gbl.columnWidths = new int[] {9999,400,9999};
gbl.rowHeights = new int[] {666,400,666};
gbc.fill = GridBagConstraints.BOTH;
gbc.gridx=1; gbc.gridy=1;
gbc.anchor = GridBagConstraints.WEST;
frame.add(jbt, gbc);
}
public static void main(String...arguments)throws Exception{
frame.setVisible(true);
}
}
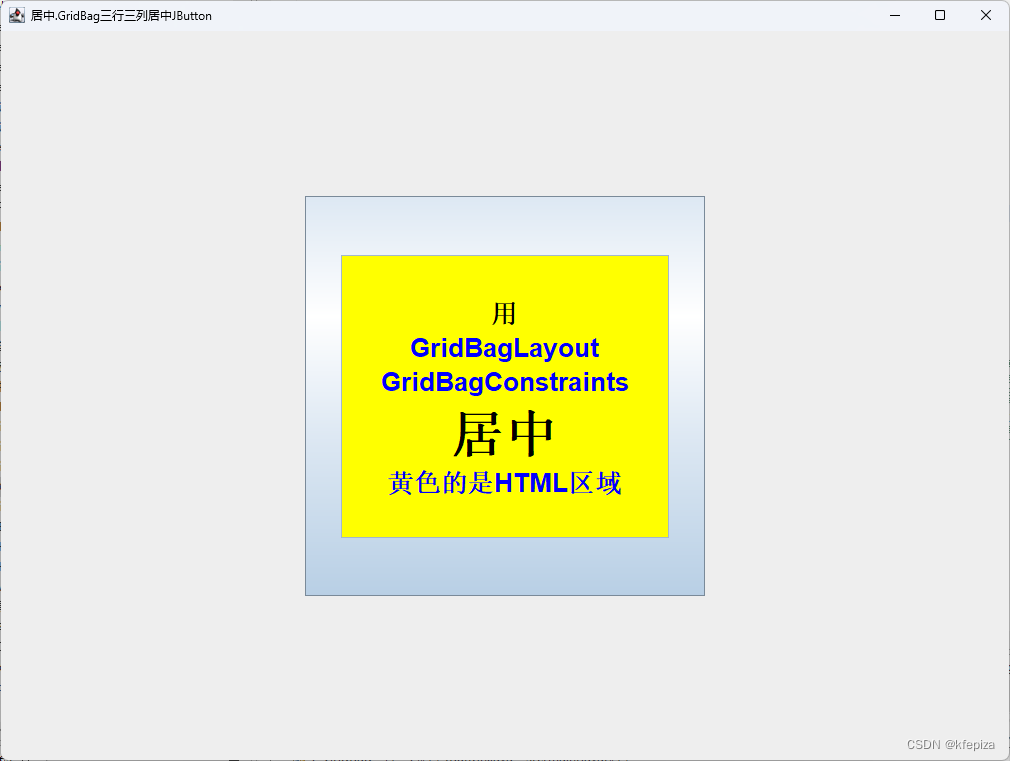