1.机械臂
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#define PORT 8888
#define IP "192.168.122.170"
int main(int argc, const char *argv[])
{
int sfd=socket(AF_INET,SOCK_STREAM,0);
if(sfd == -1)
{
perror("open socket");
return -1;
}
int reuse=1;
if(setsockopt(sfd, SOL_SOCKET, SO_REUSEADDR, &reuse, sizeof(reuse)) ==-1 )
{
perror("setsockopt");
return -1;
}
struct sockaddr_in client;
client.sin_family = AF_INET;
client.sin_addr.s_addr = inet_addr(IP);
client.sin_port = htons(PORT);
if(connect(sfd,(struct sockaddr*)&client, sizeof(client)) == -1)
{
perror("open connect");
return -1;
}
char red_buf[5]={0xff, 0x02, 0x00, 0x00, 0xff};
unsigned char blue_buf[5]={0xff, 0x02, 0x01, 0x00, 0xff};
char value;
while(1)
{
value=getchar();
if(value == '#')
{
printf("您已经退出\n");
break;
}
switch(value)
{
case 'w':
case 'W':
if(red_buf[3]>90)
{
red_buf[3] =90;
send(sfd, red_buf, sizeof(red_buf), 0);
break;
}
red_buf[3] +=5;
send(sfd, red_buf, sizeof(red_buf), 0);
break;
case 's':
case 'S':
if(red_buf[3]< -90)
{
red_buf[3]=-90;
send(sfd, red_buf, sizeof(red_buf), 0);
break;
}
red_buf[3] -=5;
send(sfd, red_buf, sizeof(red_buf), 0);
break;
case 'a':
case 'A':
if(blue_buf[3]<0)
{
blue_buf[3] =0;
send(sfd, blue_buf, sizeof(blue_buf), 0);
break;
}
blue_buf[3] -=5;
send(sfd, blue_buf, sizeof(blue_buf), 0);
break;
case 'd':
case 'D':
if(blue_buf[3]>180)
{
blue_buf[3] =180;
send(sfd, blue_buf, sizeof(blue_buf), 0);
break;
}
blue_buf[3] +=5;
send(sfd, blue_buf, sizeof(blue_buf), 0);
break;
}
}
return 0;
}
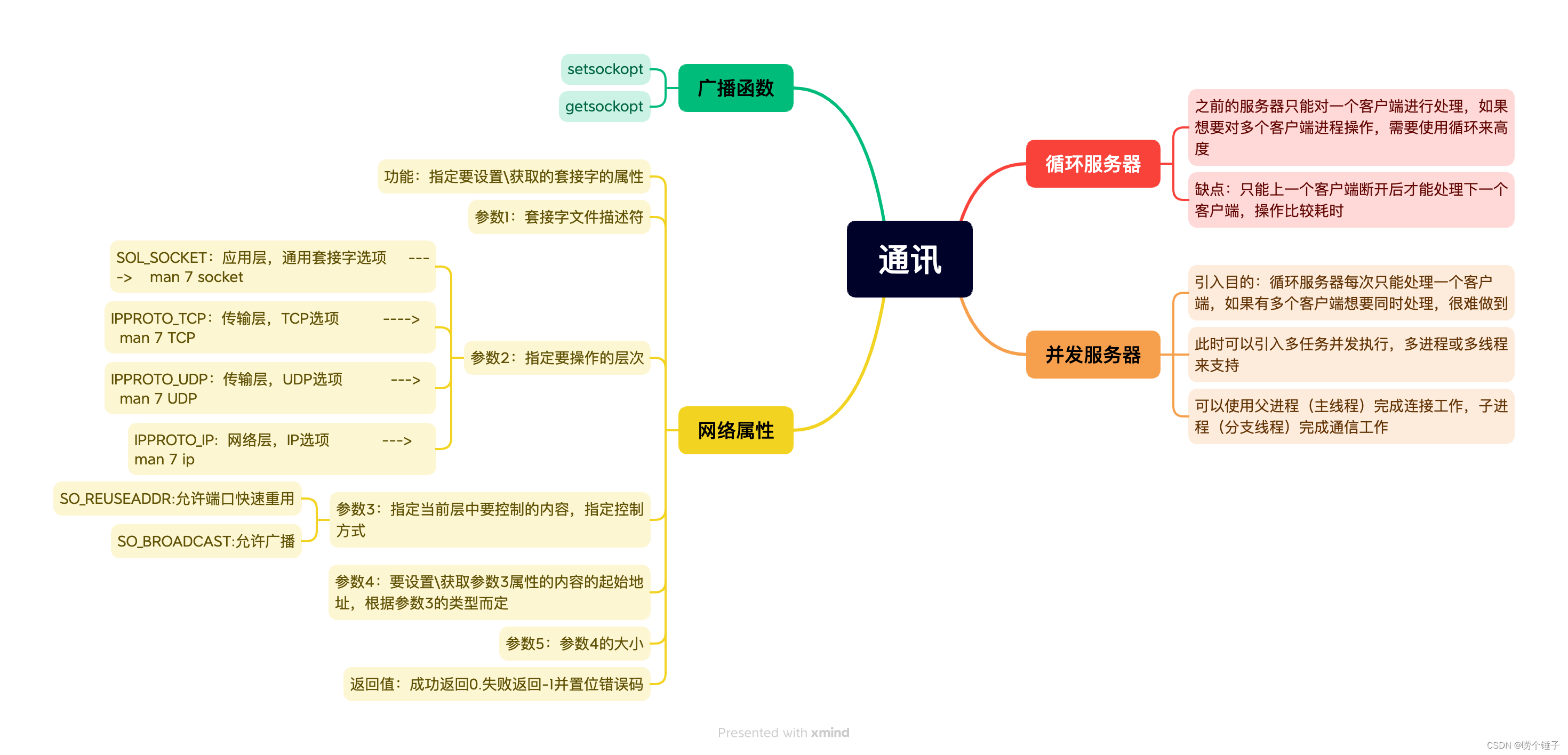