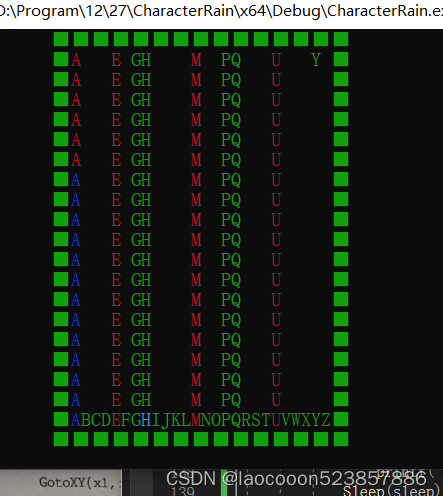
// CharacterRain.c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
int state[26];
int sleep = 100;//[100到1000]之间
int fcolor = 1;
int bcolor = BACKGROUND_GREEN;
int x1 = 10, y1 = 1, x2 = 10 + 26 + 2, y2 = 1 + 20;
void GotoXY(int x, int y) //将光标移动到(x,y)位置
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE); //获取标准输出设备句柄
COORD pos = { x-1,y-1 }; //坐标位置
SetConsoleCursorPosition(handle, pos); //设置控制台光标位置
}
void HideCursor()
{
CONSOLE_CURSOR_INFO cursor_info = { 1,0 }; //0表示隐藏光标
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
}
void DrawRectangle(int x, int y, int x2, int y2)
{
int i;
for (i = x; i <=x2; i = i + 2)
{
GotoXY(i, y);
printf("■");
GotoXY(i, y2);
printf("■");
}
for (i = y; i < y2; i = i + 1)
{
GotoXY(x, i);
printf("■");
GotoXY(x2, i);
printf("■");
}
}
void Do() {
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
// 获取控制台屏幕缓冲区信息
CONSOLE_SCREEN_BUFFER_INFO consoleInfo;
if (GetConsoleScreenBufferInfo(hConsole, &consoleInfo))
{
// 设置控制台缓冲区大小
COORD bufferSize = {
consoleInfo.srWindow.Right - consoleInfo.srWindow.Left + 1,
consoleInfo.srWindow.Bottom - consoleInfo.srWindow.Top + 1
};
SetConsoleScreenBufferSize(hConsole, bufferSize);
// 设置文本属性
SetConsoleTextAttribute(hConsole, bcolor | fcolor);
// 清空屏幕并输出黄色的区域
system("cls");
for (int i = 0; i < bufferSize.Y; i++)
{
for (int j = 0; j < bufferSize.X; j++)
{
printf(" ");
}
printf("\n");
}
// 恢复原有文本属性
SetConsoleTextAttribute(hConsole, consoleInfo.wAttributes);
}
}
void change() {
if (kbhit())
{
char ch = getch();
if (ch == 'a') //改色
{
fcolor += 1;
fcolor %= 4;
if (!fcolor)
fcolor = 1;
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), fcolor);
}
else if (ch == 'q') {
exit(0);
}
else if (ch == 'b') {//减速
sleep *= 2;
sleep = sleep > 1000 ? 1000 : sleep;
}
else if (ch == 'c') {//加速
sleep /= 2;
sleep = sleep == 0 ? 100 : sleep;
}
else if (ch == 'd') {//背景色
fcolor = fcolor == BACKGROUND_GREEN ? BACKGROUND_BLUE : fcolor;
Do();
DrawRectangle(x1, y1, x2, y2);
GotoXY(x1 + 2, y2 - 1);
printf("ABCDEFGHIJKLMNOPQRSTUVWXYZ");
}
}
}
int main()
{
int i;
char c;
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE),FOREGROUND_GREEN);
system("mode con cols=80 lines=22");
HideCursor(0);
DrawRectangle(x1, y1, x2, y2);
GotoXY(x1 + 2, y2 - 1);
printf("ABCDEFGHIJKLMNOPQRSTUVWXYZ");
do
{
c = rand() % 26 + 'A';
state[c - 'A'] = (state[c - 'A']==0?1:0);
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), fcolor + state[c - 'A']);
for (i = y1 + 1; i < y2 - 1; i = i + 1)
{
change();
GotoXY(x1 + c - 'A' + 2, i);
printf("%c", c);
Sleep(sleep);
change();
GotoXY(x1 + c - 'A' + 2, i);
printf("");
}
GotoXY(x1 + c - 'A' + 2, i);
printf("=");
Sleep(200);
GotoXY(x1 + c - 'A' + 2, i);
printf("%c", c);
} while (1);
return 0;
}