Day1
思维导图
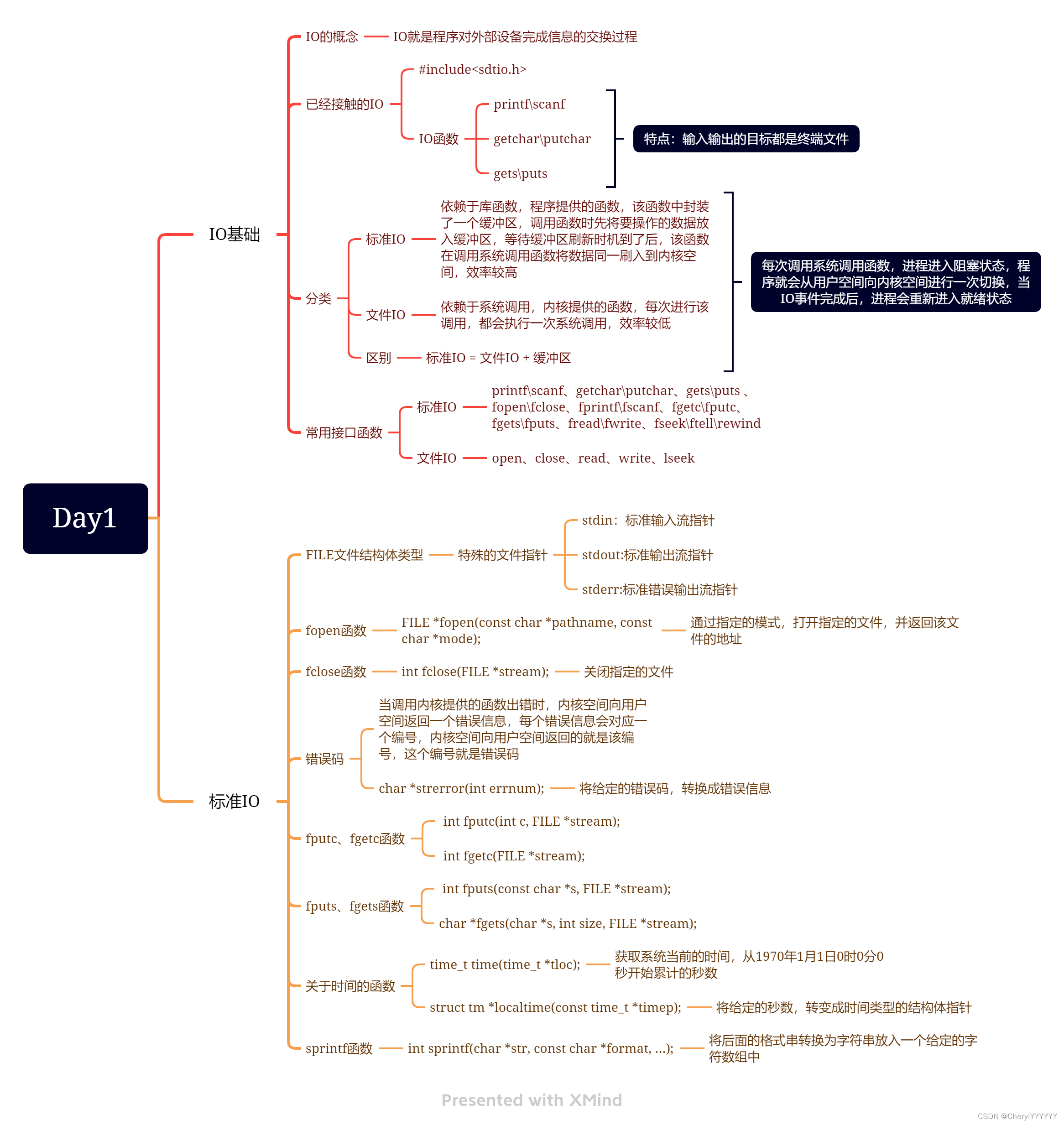
作业
使用fgets统计一个文件的行号
代码
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, const char *argv[])
{
FILE *fp=NULL;
fp=fopen("./1.c","r");
char buf=0;
int count=0;
while(1)
{
buf=fgetc(fp);
if(buf == '\n')
count++;
if(buf==EOF)
break;
}
printf("%d\n",count);
fclose(fp);
return 0;
}
运行结果

使用fgets、fputs完成两个文件的拷贝
代码
#include<stdio.h>
int main(int argc, const char *argv[])
{
if(argc != 3)
{
printf("input file error\n");
printf("usage:./a.out srcfile destfile\n");
return 0;
}
FILE *srcfp, *destfp;
if((srcfp = fopen(argv[1], "r")) == NULL)
{
perror("srcfile open error");
return -1;
}
if((destfp = fopen(argv[2], "w")) == NULL)
{
perror("destfile open error");
return -1;
}
char buf = 0;
while((buf = fgetc(srcfp)) != EOF)
{
fputc(buf, destfp);
}
fclose(srcfp);
fclose(destfp);
printf("拷贝成功\n");
return 0;
}
运行结果
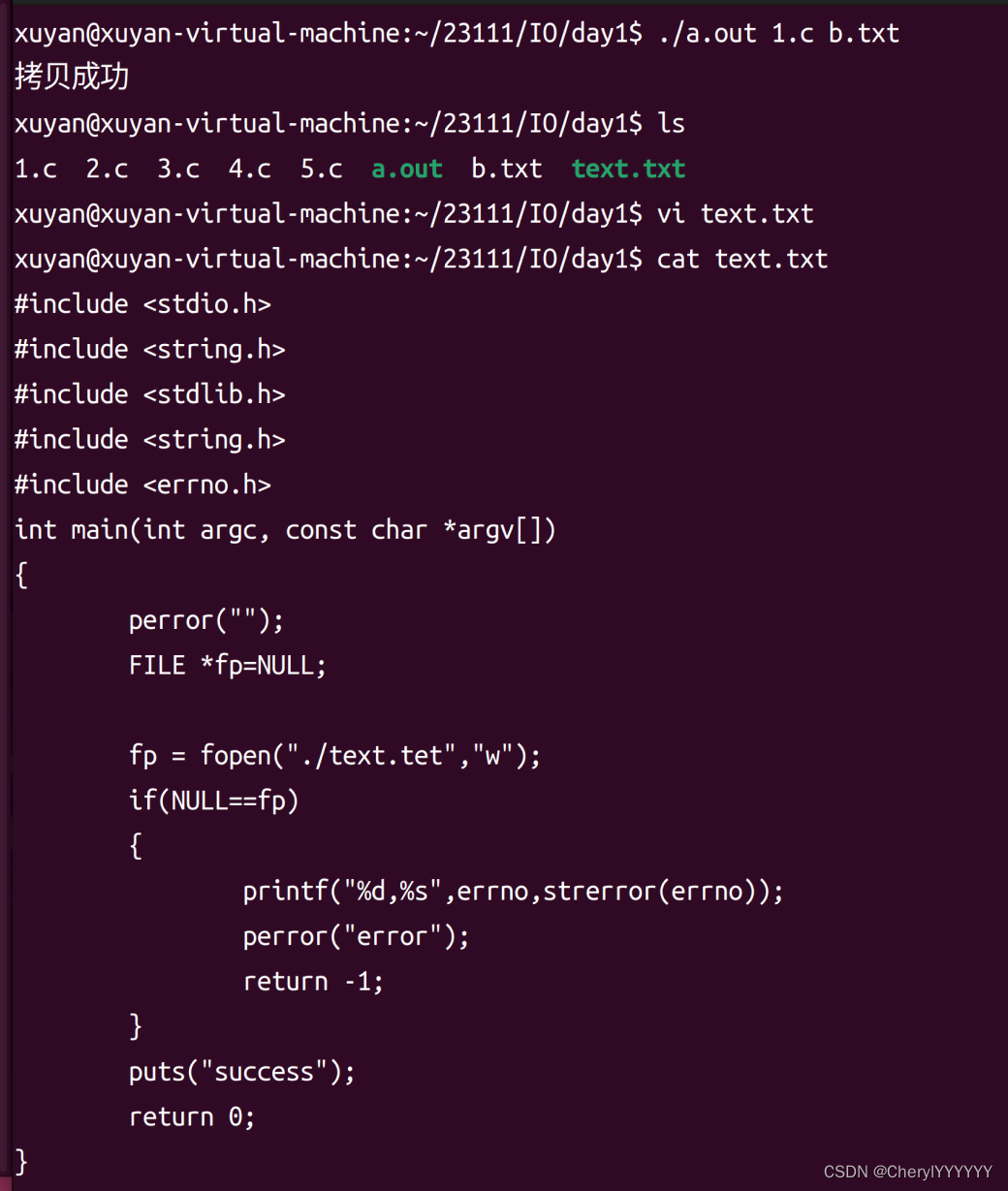
向文件中输出当前的系统时间
代码
#include<stdio.h>
#include<time.h>
int main(int argc, const char *argv[])
{
while(1)
{
time_t sys_time = 0;
time(&sys_time);
struct tm* t = localtime(&sys_time);
char buf[128] ="";
sprintf(buf,"%4d-%02d-%02d %02d:%02d:%02d",t->tm_year+1900, t->tm_mon+1,\
t->tm_mday, t->tm_hour, t->tm_min, t->tm_sec);
printf("buf = %s\n", buf);
sleep(1);
}
return 0;
}
运行结果
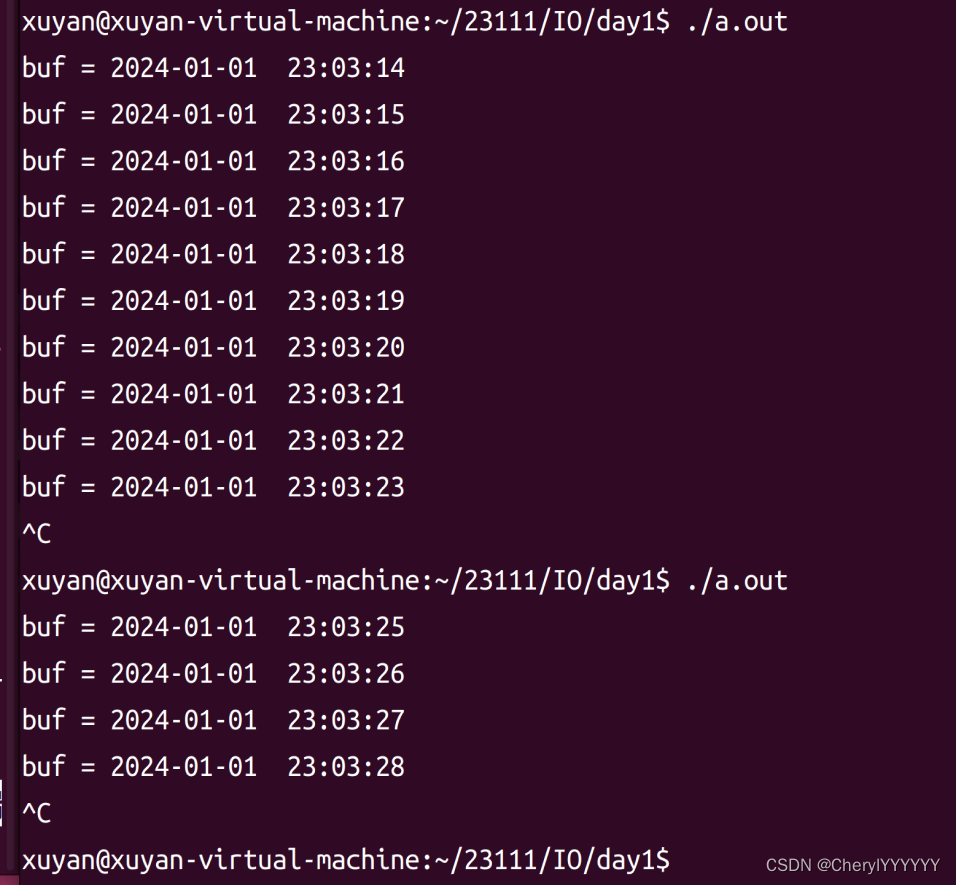