定义一个Person类,包含私有成员,int *age,string &name,一个Stu类,包含私有成员double *score,Person p1,写出Person类和Stu类的特殊成员函数,并写一个Stu的show函数,显示所有信息。 #include <iostream>
using namespace std;
class Person
{
int *age;
string &name;
public:
int get_age();
string & get_name();
Person(int a,string &name):age(new int(a)),name(name) {cout<<"Person的有参构造函数"<<endl;}
Person(const Person &other):age(new int (*(other.age))),name(other.name)
{
cout<<"Person的拷贝构造函数"<<endl;
}
Person &operator=(const Person &other)
{
*(this->age)=*(other.age);
this->name=(other.name);
cout<<"Person的拷贝赋值函数"<<endl;
return *this;
}
~Person()
{
delete age;
cout<<"Person的析构函数"<<endl;
}
};
class Stu
{
double *score;
Person p1;
public:
void set(int a,int b);
void show();
Stu(double a,Person b):score(new double(a)),p1(b)
{
cout<<"Stu的有参构造函数"<<endl;
}
Stu(const Stu &other):score(new double (*(other.score))),p1(other.p1)
{
cout<<"Stu的拷贝构造函数"<<endl;
}
Stu &operator=(const Stu &other)
{
*(this->score)=*(other.score);
this->p1=(other.p1);
cout<<"Stu的拷贝赋值函数"<<endl;
return *this;
}
~Stu()
{
delete score;
cout<<"Stu的析构函数"<<endl;
}
};
int Person::get_age()
{
return *age;
}
string & Person::get_name()
{
return name;
}
void Stu::show()
{
cout<<"age="<<p1.get_age()<<endl;
cout<<"name="<<p1.get_name()<<endl;
cout<<"score="<<*score<<endl;
}
int main()
{
string n="zhangsan";
Person a(18,n);
Stu s(99,a);
s.show();
Person b(20,n);
return 0;
}
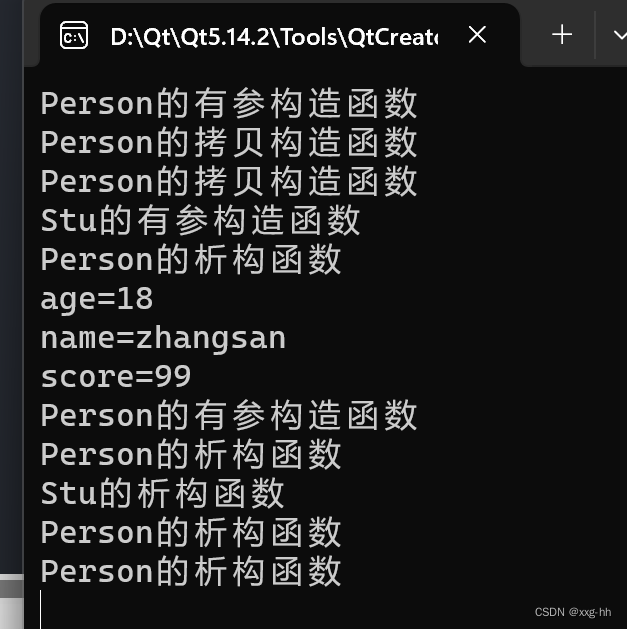