栈实现队列
实现方式:
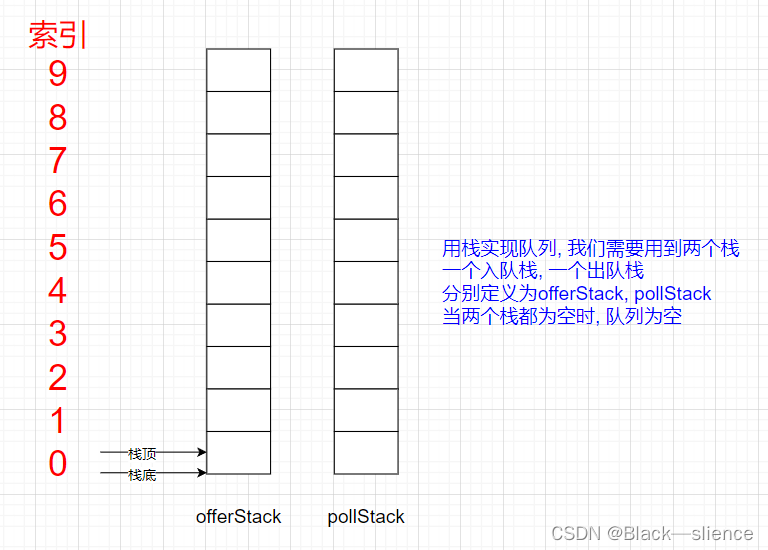
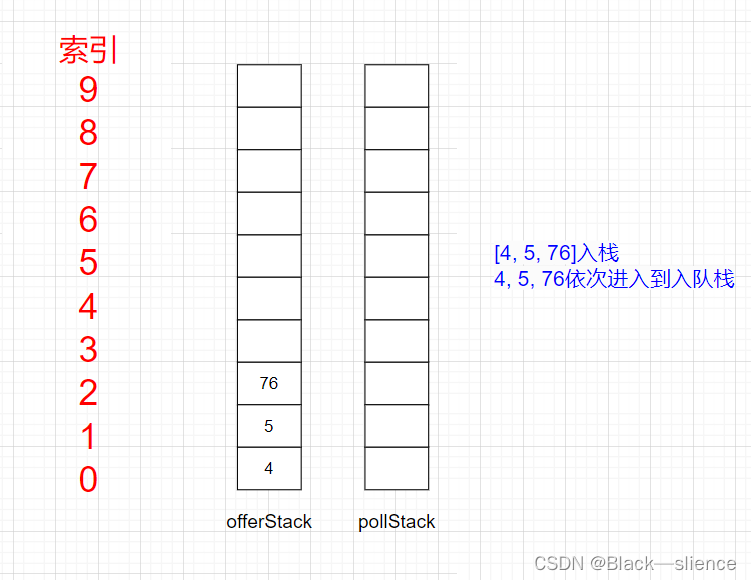
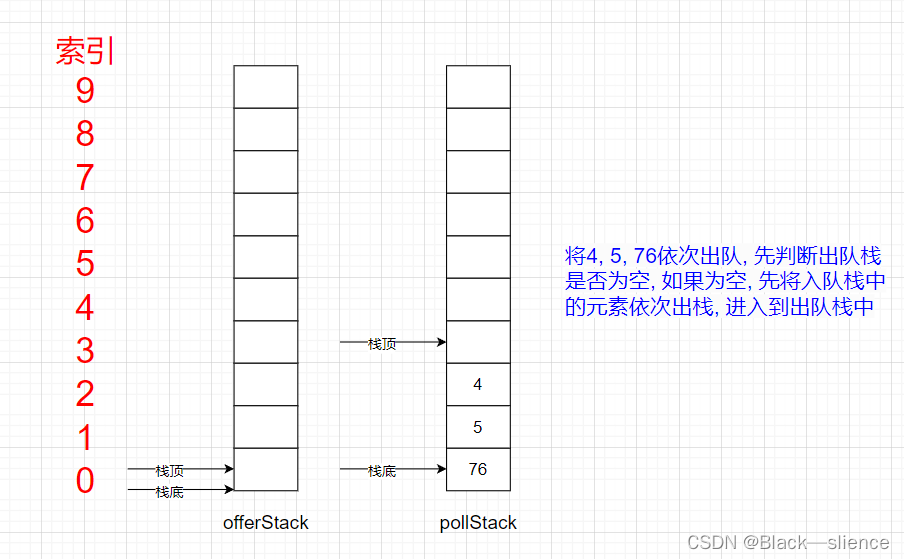
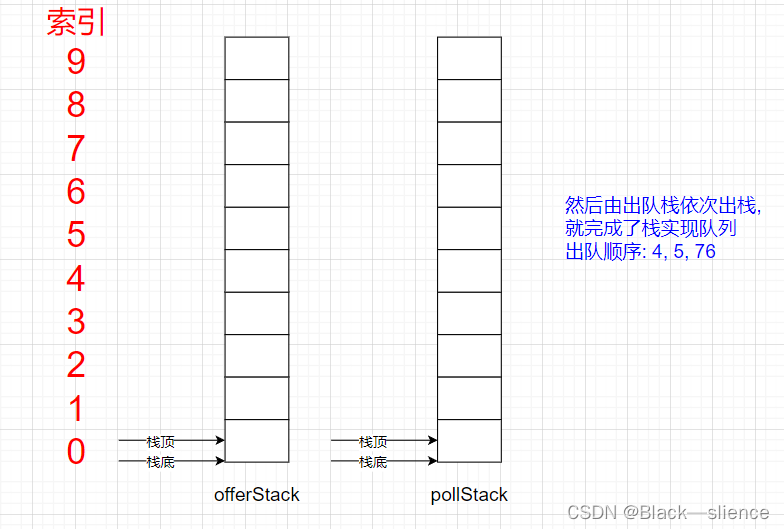
代码:
public class MyQueue {
private Stack<Integer> offerStack;// 入队栈
private Stack<Integer> pollStack;// 出队栈
public MyQueue() {
offerStack = new Stack<>();
pollStack = new Stack<>();
}
// 入队
public void offer(int x) {
offerStack.push(x);
}
// 出队
public int poll() {
if (!empty()) {
if (pollStack.empty()) {
offerToPoll();
}
return pollStack.pop();
}
return -1;
}
// 入队栈向出队栈转移元素
private void offerToPoll() {
if (!offerStack.empty()) {
int size = offerStack.size();
for (int i = 0; i < size; i++) {
pollStack.push(offerStack.pop());
}
}
}
// 返回队头的元素
public int peek() {
if (!empty()) {
if (pollStack.empty()) {
offerToPoll();
}
return pollStack.peek();
}
return -1;
}
// 判断队列是否为空
public boolean empty() {
return offerStack.empty() && pollStack.empty();
}
}
队列实现栈
实现方式: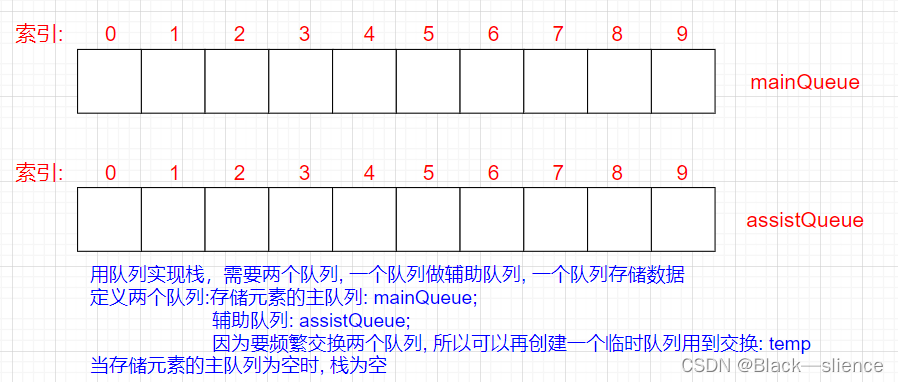
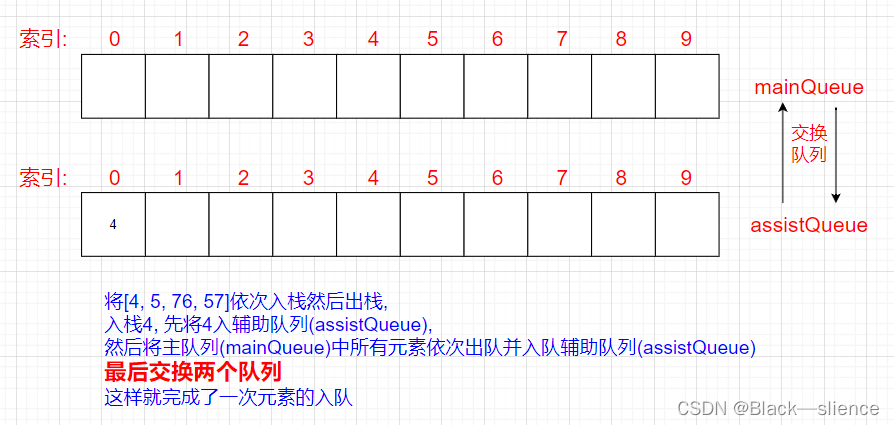
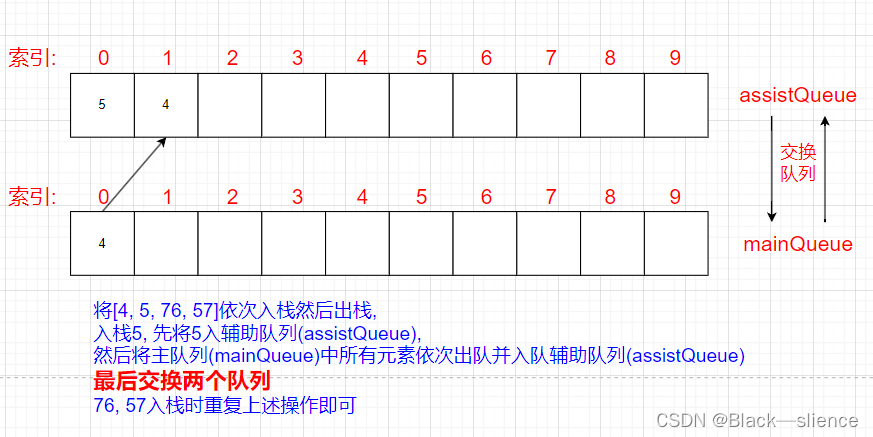
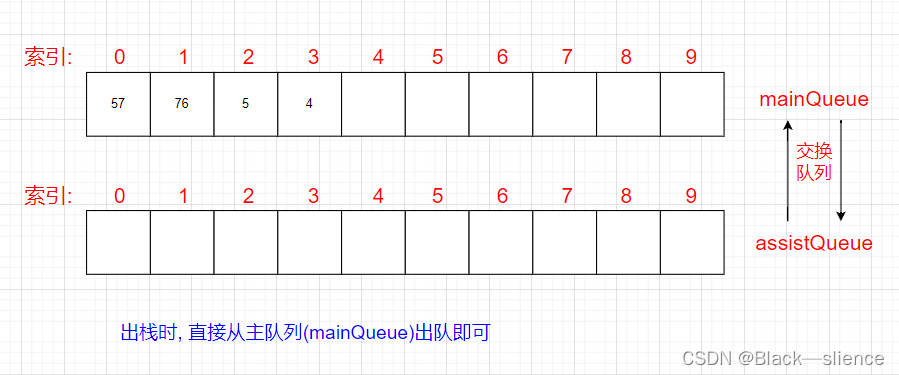
代码:
public class MyStack {
private LinkedList<Integer> mainQueue;// 主队列
private LinkedList<Integer> assistQueue;// 辅助队列
private LinkedList<Integer> temp;// 交换两个队列的中间变量
public MyStack() {
mainQueue = new LinkedList<>();
assistQueue = new LinkedList<>();
temp = null;
}
// 入栈
public void push(int x) {
assistQueue.offer(x);
while (!mainQueue.isEmpty()) {
assistQueue.offer(mainQueue.poll());
}
temp = assistQueue;
assistQueue = mainQueue;
mainQueue = temp;
}
// 出栈
public int pop() {
Integer result = null;
if (!mainQueue.isEmpty()) {
result = mainQueue.poll();
}
return result;
}
// 查看栈顶元素
public int peek() {
Integer result = null;
if (!mainQueue.isEmpty()) {
result = mainQueue.peek();
}
return result;
}
// 判断栈是否为空
public boolean empty() {
return mainQueue.isEmpty();
}
}