定义一个Person类,私有成员int age,string &name,定义一个Stu类,包含私有成员double *score,写出两个类的构造函数、析构函数、拷贝构造和拷贝赋值函数,完成对Person的运算符重载(算术运算符、条件运算符、逻辑运算符、自增自减运算符、插入/提取运算符) //主程序
#include"stuper.h"
int main()
{
cout<<boolalpha<<endl;
string name="lisi";
//Person p;
Person p1(23,18,name);//自动调用有参构造
p1.show();
Person p2=p1;//自动调用拷贝构造函数
p2.show();
Person p3(p2);//自动调用拷贝构造函数
p3.show();
Person q1(10,20,name);
Person q2(6,15,name);
Person q3=q1+q2;
q3.show1();
//插入<<运算符重载
cout<<"p1:"<<p1<<endl;
if(q1>q2)
{
cout<<"q1>q2"<<endl;
}
q1=q2++;
q2.show();
q1.show();
Person p5;
cin>>p5;
cout<<"p5:"<<p5<<endl;
cout<<"============================================"<<endl;
Stu s1;//自动调用无参构造
Stu s2(88);//自动调用有参构造
s2.show();
Stu s3=s2;//自动调用拷贝构造函数
s3.show();
Stu s4(s3);//自动调用拷贝构造函数
s4.show();
s1=s2;
s1.show();
return 0;
}
//头文件
#ifndef STUPER_H
#define STUPER_H
#include <iostream>
using namespace std;
class Person;
const Person operator++(Person &O,int);
ostream &operator<<(ostream &cout, const Person &p);
istream &operator>>(istream &cin, Person &p);
class Person
{
friend istream &operator>>(istream &cin, Person &p);
friend const Person operator++(Person &O,int);
friend ostream &operator<<(ostream &cout, const Person &p);
private:
int age;
int age1;
string n="df";
string &name=n;
public:
//无参构造
Person(){cout<<"Person无参构造"<<endl;}
//有参构造
Person(int age,int age1,string &name):age(age),age1(age1),name(name)
{
cout<<"Person有参构造"<<endl;
}
//析构函数
~Person()
{
cout << "Person::析构函数" << endl;
}
//拷贝构造函数
Person(const Person &other):age(other.age),age1(other.age1),name(other.name)
{
cout << "Person::拷贝构造函数" << endl;
}
//拷贝赋值函数
Person &operator=(const Person &other)
{
if(this != &other) //避免自己赋值给自己
{
age = other.age; //深拷贝
age1=other.age1;
name=other.name;
}
cout << "拷贝赋值函数" << endl;
return *this;
}
//输出
void show()
{
cout<<"age="<<age<<" age1="<<age1<<" name="<<name<<endl;
}
void show1()
{
cout<<"age="<<age<<" age1="<<age1<<endl;
}
//Person的运算符重载
//算术运算符
//成员函数实现+号运算符重载
const Person operator+(const Person &R) const
{
Person temp;
temp.age = this->age + R.age;
temp.age1= this->age1 + R.age1;
return temp;
}
//局部函数实现>号运算符重载
bool operator>( const Person &R)const
{
if(age > R.age && age1 > R.age1)
{
return true;
}
else
{
return false;
}
}
};
//==========================================
class Stu
{
private:
double *score;
public:
//无参构造
Stu(){cout<<"Stu无参构造"<<endl;}
//有参构造
Stu(double score):score(new double(score))
{
cout<<"Stu有参构造"<<endl;
}
//析构函数
~Stu()
{
cout << "Stu::析构函数" << endl;
delete score;
}
//拷贝构造函数
Stu(const Stu &other):score(new double(*(other.score)))
{
cout << "Stu::拷贝构造函数" << endl;
}
//拷贝赋值函数
Stu &operator=(const Stu &other)
{
if(this != &other) //避免自己赋值给自己
{
score = new double(*(other.score)); //深拷贝
}
cout << "拷贝赋值函数" << endl;
return *this;
}
void show()
{
cout<<"score="<<*score<<endl;
}
};
#endif // STUPER_H
//函数体
#include "stuper.h"
//全局函数实现前置++运算符重载
const Person operator++(Person &O,int)
{
Person temp;
temp.age=O.age++;
temp.age1=O.age1++;
return temp;
}
//全局函数实现插入<<运算符重载
ostream &operator<<(ostream &cout, const Person &p)
{
cout << "age = " << p.age << " age1 = " << p.age1<<"name = "<<p.name;
return cout;
}
//全局函数实现提取>>运算符重载
istream &operator>>(istream &cin, Person &p)
{
cin >> p.age >> p.age1>>p.name;
return cin;
}
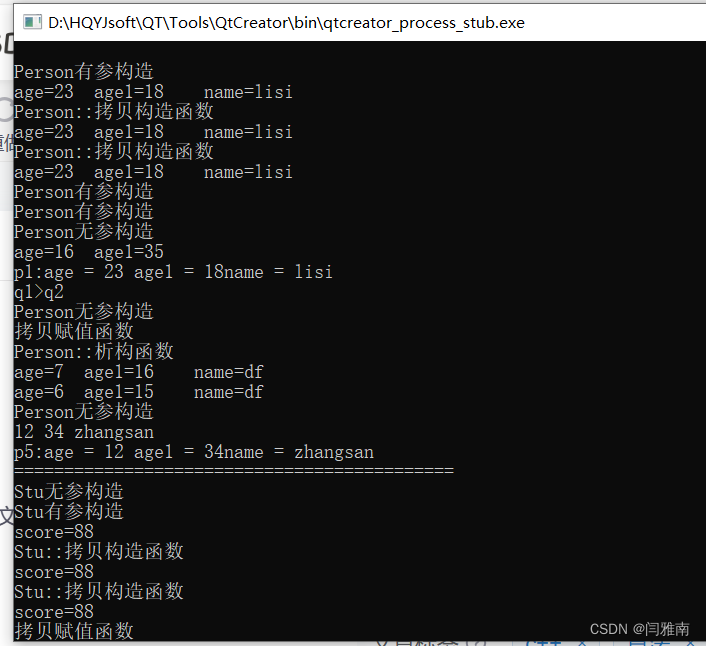
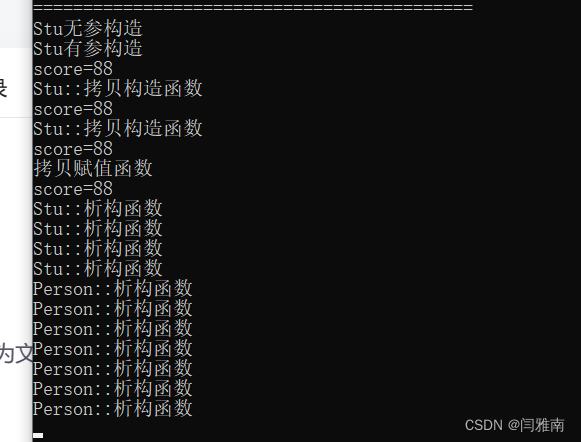