微信公众号接入指南文档
https://developers.weixin.qq.com/doc/offiaccount/Basic_Information/Access_Overview.html
在微信公众号的服务器配置中,首先需要编写Java代码来处理微信服务器的验证请求。以下是Java示例代码,然后我将为你提供启用服务器配置的步骤。(点击启用后微信服务器会调用该接口验证)
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Arrays;
@Slf4j
@RestController
@RequestMapping("/wx")
public class WeChatController {
// 替换成你在微信公众平台设置的Token
private static final String TOKEN = "your_wechat_token";
/**
* 验证消息的确来自微信服务器
*
* @param signature 微信加密签名,signature结合了开发者填写的token参数和请求中的timestamp参数、nonce参数
* @param timestamp 时间戳
* @param nonce 随机数
* @param echostr 随机字符串
* @return
*/
@RequestMapping(value = "/validateWeChatServer", method = RequestMethod.GET)
public String validateWeChatServer(@RequestParam(name = "signature") String signature,
@RequestParam(name = "timestamp") String timestamp,
@RequestParam(name = "nonce") String nonce,
@RequestParam(name = "echostr") String echostr) {
// 将参数按字典序排序
String[] params = {TOKEN, timestamp, nonce};
Arrays.sort(params);
// 拼接参数并进行SHA1加密
String encrypted = sha1Encode(params[0] + params[1] + params[2]);
// 校验签名
if (encrypted.equals(signature)) {
// 校验通过,返回echostr参数
log.info("验证通过,消息的确来自微信服务器, 返回echostr参数: {}", echostr);
return echostr;
} else {
// 校验失败,返回错误信息
return "Invalid signature";
}
}
private String sha1Encode(String input) {
try {
MessageDigest digest = MessageDigest.getInstance("SHA-1");
byte[] hash = digest.digest(input.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
return null;
}
}
}
在上述代码中,/your_wechat_token是你在微信公众平台设置的Token。这个接口用于验证微信服务器配置的有效性。
接下来是如何启用服务器配置的步骤:
1. 登录微信公众平台,进入公众号后台。
2. 在左侧菜单中选择【开发】->【基本设置】。
3. 找到【服务器配置】,点击右侧的【修改配置】按钮。
4. 填入你的服务器配置信息:
URL(服务器地址): 填写你的服务器地址,例如 https://xxx/wx/validateWeChatServer
Token: 填写你在Java代码中设置的Token。
EncodingAESKey: 如果你的消息加解密方式选择的是安全模式,需要填写。
5. 将Java代码部署到你的服务器上,确保服务器可以被外部访问。
6. 点击【保存并测试】,如果配置正确,你会看到【配置成功】的提示。
7. 确认配置无误后,点击【启用】按钮,使服务器配置生效。
完成上述步骤后,你的Java代码就能处理微信服务器的验证请求,服务器配置也会启用。接下来,你可以在代码中处理用户消息等业务逻辑。
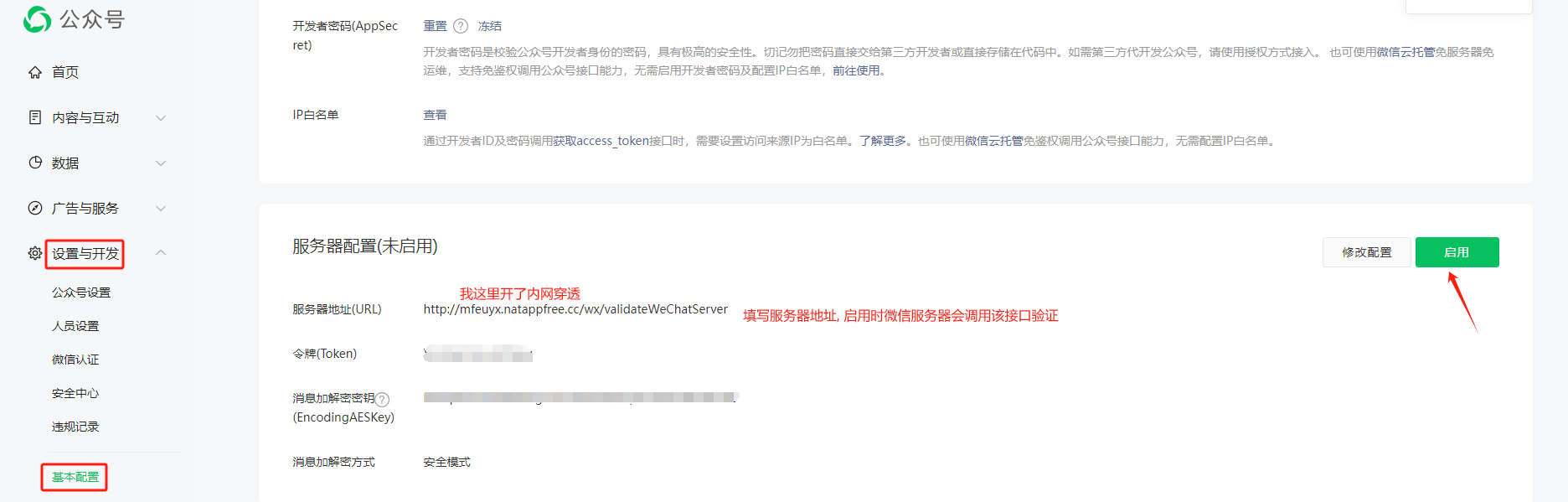