#include <iostream>
using namespace std;
class Time
{
private:
int h, m, s;
public:
// 构造函数
Time(int hours = 0, int minutes = 0, int seconds = 0) : h(hours), m(minutes), s(seconds) {}
// 重载加法运算符
Time operator+(const Time &other) const
{
Time result;
result.s = s + other.s;
result.m = m + other.m + result.s / 60;
result.h = h + other.h + result.m / 60;
result.s %= 60;
result.m %= 60;
return result;
}
// 重载减法运算符
Time operator-(const Time &other) const
{
Time result;
result.s = s - other.s;
result.m = m - other.m;
result.h = h - other.h;
// 处理借位
if (result.s < 0)
{
result.s += 60;
result.m--;
}
if (result.m < 0)
{
result.m += 60;
result.h--;
}
return result;
}
// 重载整数加法运算符
Time operator+(int seconds) const
{
Time result = *this;
result.s += seconds;
result.m += result.s / 60;
result.h += result.m / 60;
result.s %= 60;
result.m %= 60;
return result;
}
// 重载输出运算符
friend std::ostream &operator<<(std::ostream &os, const Time &time)
{
os << time.h << "时" << time.m << "分" << time.s << "秒";
return os;
}
// 重载输入运算符
friend std::istream &operator>>(std::istream &is, Time &time)
{
is >> time.h >> time.m >> time.s;
return is;
}
// 扩展:通过下标取出时分秒
int &operator[](int index)
{
if (index == 0)
return h;
else if (index == 1)
return m;
else if (index == 2)
return s;
// 可以根据实际需要扩展其他下标的处理
}
};
int main()
{
Time t1(1, 30, 45);
Time t2(0, 45, 30);
// 使用运算符重载
Time sum = t1 + t2;
Time dif = t1 - t2;
Time read = t1 + 120;
// 输出结果
std::cout << "t1: " << t1 << std::endl;
std::cout << "t2: " << t2 << std::endl;
std::cout << "Sum: " << sum << std::endl;
std::cout << "dif: " << dif << std::endl;
std::cout << "read: " << read << std::endl;
// 输入时间
Time t3;
std::cout << "输入时间 (hh mm ss): ";
std::cin >> t3;
std::cout << "t3时间: " << t3 << std::endl;
// 使用扩展的下标操作符
std::cout << "ti h: " << t1[0] << std::endl;
std::cout << "t1 min: " << t1[1] << std::endl;
std::cout << "t1 s: " << t1[2] << std::endl;
return 0;
}
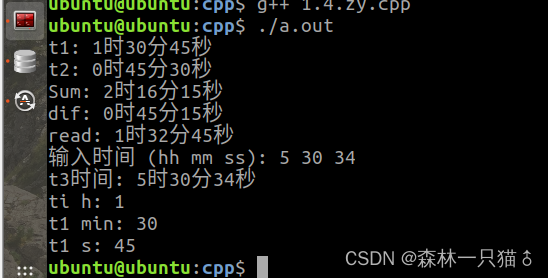