1、FileZilla设置
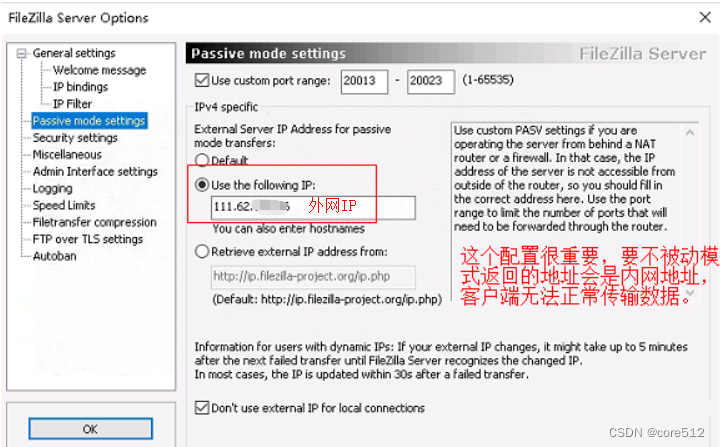
2、java代码实现
package src;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.text.SimpleDateFormat;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import org.apache.commons.net.ftp.FTPReply;
public class TestFTPClient
{
public static void main(String[] args) throws Exception
{
FTPClient ftp = new FTPClient();
ftp.setConnectTimeout(30000);
ftp.setDataTimeout(30000);
ftp.connect("1.1.1.1", 1025);
int reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply))
{
ftp.disconnect();
System.out.println("** 无法连接至FTP服务器!");
System.exit(1);
}
if (!ftp.login("abc", "abc***"))
{
ftp.logout();
System.out.println("** 错误的用户名或密码!");
System.exit(1);
}
System.out.println("Connected.");
ftp.setFileType(FTP.BINARY_FILE_TYPE);
ftp.enterLocalPassiveMode();
ftp.setControlEncoding("UTF-8");
listFile(ftp);
ftp.logout();
ftp.disconnect();
System.out.println("Quit.");
}
public static void upload(FTPClient ftp)
{
File localFile = new File("D:\\test121.txt");
InputStream inStream;
OutputStream outStream;
try
{
String remotePath = ftpPath(localFile.getName());
inStream = new FileInputStream(localFile);
outStream = ftp.storeFileStream(remotePath);
byte[] buffer = new byte[4000];
while (true)
{
int n = inStream.read(buffer);
if (n <= 0)
break;
outStream.write(buffer, 0, n);
}
inStream.close();
outStream.close();
} catch (FileNotFoundException e1)
{
e1.printStackTrace();
} catch (IOException e)
{
e.printStackTrace();
}
}
public static void listFile(FTPClient ftp)
{
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
System.out.println("> list");
FTPFile[] ftpFiles;
try
{
ftpFiles = ftp.listFiles();
for (FTPFile f : ftpFiles)
{
String time = sdf.format(f.getTimestamp().getTime());
String info = "";
if (f.isDirectory())
info = String.format("+ %-20s", f.getName());
else
info = String.format(" %-20s %8d %s", f.getName(), f.getSize(), time);
System.out.println(info);
}
} catch (IOException e)
{
e.printStackTrace();
}
}
public static String ftpPath(String path)
{
try
{
return new String(path.getBytes("UTF-8"), FTP.DEFAULT_CONTROL_ENCODING);
} catch (UnsupportedEncodingException e)
{
return "";
}
}
public static void download(FTPClient ftp) throws Exception
{
File localFile = new File("D:/tmp/copy.zip");
localFile.getParentFile().mkdirs();
String remotePath = ftpPath("/HBiuder.zip");
OutputStream outStream = new FileOutputStream(localFile);
InputStream inStream = ftp.retrieveFileStream(remotePath);
if(inStream == null)
throw new Exception("远程文件不存在!" + remotePath);
byte[] buffer = new byte[4000];
while(true)
{
int n =inStream.read(buffer);
if(n <= 0) break;
outStream.write(buffer,0,n);
}
inStream.close();
outStream.close();
}
}