1、用数据结构定义学生信息,有学号,姓名,5门课程的成绩,编一程序,输入20个学生成绩,求出总分最高的学生姓名并输出结果。要求编写3个函数,它们的功能分别为:
1)输入函数,用于从键盘读入学号、姓名和5门课的成绩;
2)计算总分函数,以计算每位学生的总分;
3)输出函数,显示每位学生的学号、总分和分数。
说明:这三个函数的形式参数均为结构体指针和整型变量,函数的类型均为void.
#include<stdio.h>
struct stu
{
?????? int b;
?????? char a[20];
?????? float one;
?????? float two;
?????? float three;
?????? float four;
?????? float five;
};
int main()
{
?????? struct stu s[20];
?????? float sum[20];
?????? for(int i=0;i<20;i++)
?????? {
????????????? scanf("%d",&s[i].b);
????????????? scanf("%s",&s[i].a);
????????????? scanf("%f",&s[i].one);
????????????? scanf("%f",&s[i].two);
????????????? scanf("%f",&s[i].three);
????????????? scanf("%f",&s[i].four);
????????????? scanf("%f",&s[i].five);
??????? sum[i]=s[i].one+s[i].two+s[i].three+s[i].four+s[i].five;
?????????????
?????? }
?????? int max=sum[0];
?????? int n=0;
?????? for(int j=1;j<20;j++)
?????? {
????????????? if(sum[j]>max)
????????????? {
???????????????????? max=sum[j];
???????????????????? n=j;
??????? }
????????????? printf("%-20d %s %f %f %f %f %f\n",s[j].b,s[j].a,s[j].one,s[j].two,s[j].three,s[j].four,s[j].five);
?????? }
??????
?????? printf("总分最高的学生:%s 成绩为:%f",s[n].a,sum[n]);
?????? return 0;
}
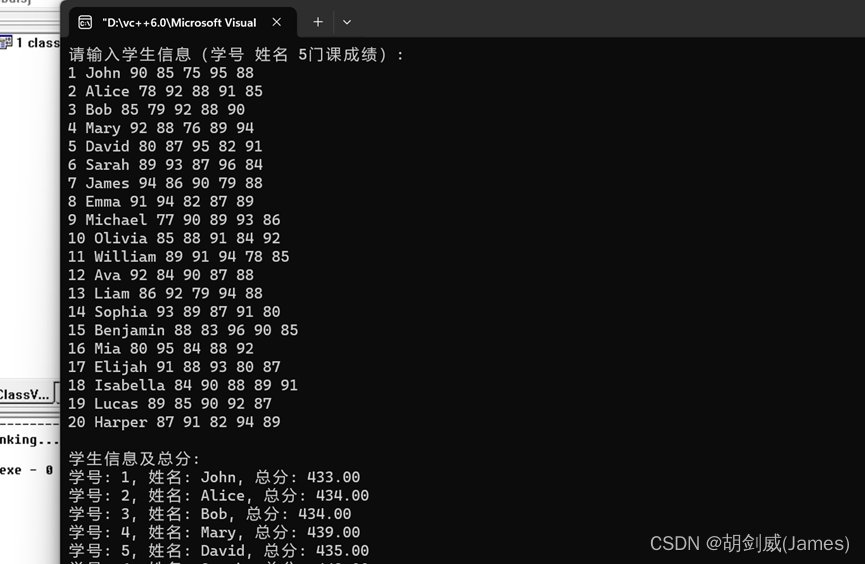
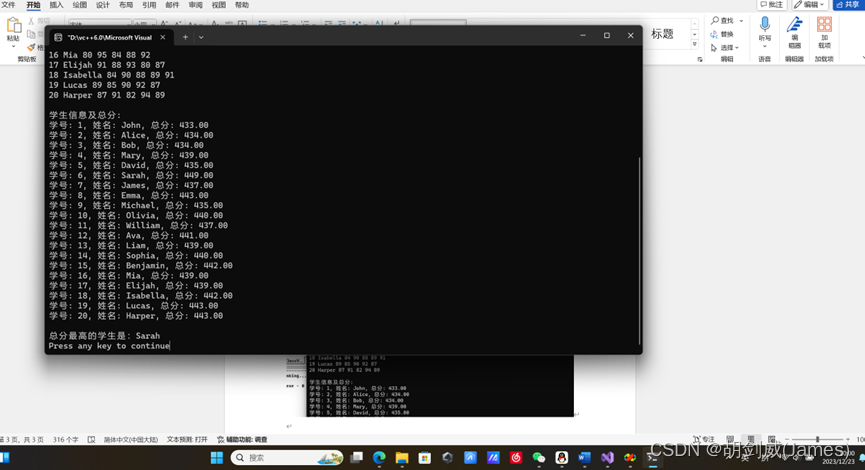
2、编写一程序,运用插入结点的方法,将键盘输入的n个整数(输入0结束)插入到链表中,建立一个从小到大的有序链表。
#include <stdio.h>
#include <stdlib.h>
struct Node {
??? int data;
??? struct Node* next;
};
struct Node* createNode(int value);
void insertSorted(struct Node** head, int value);
void displayList(struct Node* head);
void freeList(struct Node* head);
int main() {
??? struct Node* head = NULL;
??? int input;
??? printf("请输入整数,以0结束输入:\n");
??? do {
??????? scanf("%d", &input);
??????? if (input != 0) {
??????????? insertSorted(&head, input);
??????? }
??? } while (input != 0);
??? printf("有序链表的内容:\n");
??? displayList(head);
??? freeList(head);
??? return 0;
}
struct Node* createNode(int value) {
??? struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
??? if (newNode == NULL) {
??????? printf("内存分配失败\n");
??????? exit(1);
??? }
??? newNode->data = value;
??? newNode->next = NULL;
??? return newNode;
}
void insertSorted(struct Node** head, int value) {
??? struct Node* newNode = createNode(value);
??? if (*head == NULL || value < (*head)->data) {
??????? newNode->next = *head;
??????? *head = newNode;
??? } else {
??????? struct Node* current = *head;
??????? while (current->next != NULL && current->next->data < value) {
??????????? current = current->next;
??????? }
??????? newNode->next = current->next;
??????? current->next = newNode;
??? }
}
void displayList(struct Node* head) {
??? struct Node* current = head;
??? while (current != NULL) {
??????? printf("%d ", current->data);
??????? current = current->next;
??? }
??? printf("\n");
}
void freeList(struct Node* head) {
??? struct Node* current = head;
??? struct Node* next;
??? while (current != NULL) {
??????? next = current->next;
??????? free(current);
??????? current = next;
??? }
}
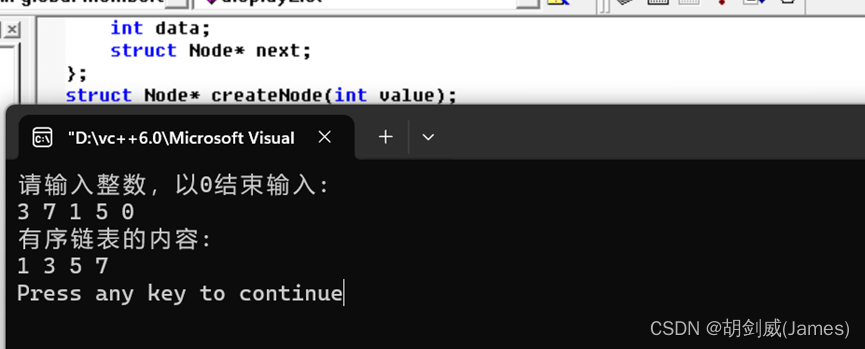