效果图
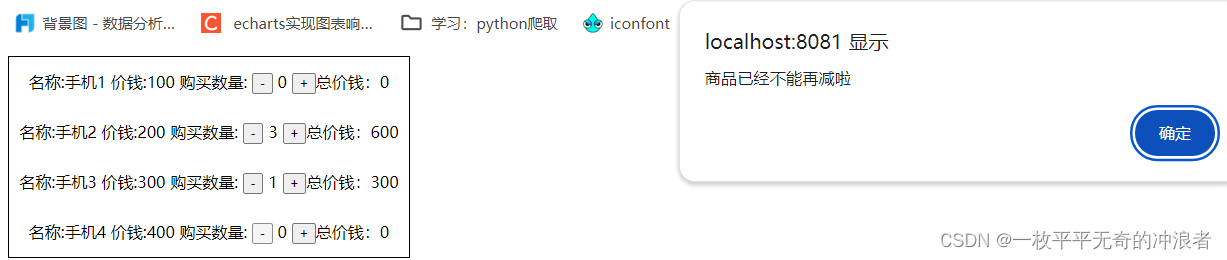
index.js文件
import Vue from "vue";
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
shopsList: [{
goodsName: "手机1",
goodsAmount: 0,
goodsPrice: 100,
totalPrice: 0,
id: 1,
},
{
goodsName: "手机2",
goodsAmount: 0,
goodsPrice: 200,
totalPrice: 0,
id: 2,
},
{
goodsName: "手机3",
goodsAmount: 0,
goodsPrice: 300,
totalPrice: 0,
id: 3,
},
{
goodsName: "手机4",
goodsAmount: 0,
goodsPrice: 400,
totalPrice: 0,
id: 4,
},
],
goodsNumber: 0
},
mutations: {
addGoodsMutations(state, goods) {
state.shopsList.forEach((item) => {
if (item.id === goods.id) {
item.goodsAmount++
}
})
},
reduceGoodsMutations(state, goods) {
if (goods.goodsAmount === 0) {
alert("商品已经不能再减啦")
return
}
state.shopsList.forEach((item) => {
if (item.id === goods.id) {
item.goodsAmount--
}
})
},
totalMutations(state, goods) {
state.shopsList.forEach((item) => {
if (item.id === goods.id) {
item.totalPrice = item.goodsAmount * item.goodsPrice
}
})
},
},
actions: {
addGoodsActions({
commit
}, item) {
commit('addGoodsMutations', item)
},
reduceGoodsActions({
commit
}, item) {
commit('reduceGoodsMutations', item)
},
totalAction({
commit
}, item) {
commit('totalMutations', item)
}
},
getters: {},
modules: {}
})
页面
<template>
<div class="box">
<div class="content" v-for="(item, index) in shopsList" :key="index">
<div>
名称:{{ item.goodsName }} 价钱:{{ item.goodsPrice }}
购买数量:
<el-button style="margin-right: 5px" @click="reduce(item)">-</el-button>
<span>{{ item.goodsAmount }}</span>
<el-button style="margin-left: 5px" @click="add(item)">+</el-button>
<span>总价钱:{{ item.totalPrice }}</span>
</div>
</div>
</div>
</template>
<script>
import { mapState, mapMutations, mapActions } from "vuex";
export default {
mounted() {},
data() {
return {};
},
computed: {
...mapState(["shopsList", "totalPrice", "goodsNumber"]),
},
methods: {
...mapMutations(["addGoodsMutations", "reduceGoodsMutations"]),
...mapActions(["addGoodsActions", "reduceGoodsActions", "totalAction"]),
reduce(item) {
this.reduceGoodsActions(item);
this.totalAction(item);
},
add(item) {
this.addGoodsActions(item);
this.totalAction(item);
},
},
};
</script>
<style>
.box {
width: 400px;
height: 200px;
border: 1px solid black;
}
.content {
height: 50px;
display: flex;
justify-content: space-evenly;
align-items: center;
}
</style>
先这样吧,后续将再完善