- 快速排序是冒泡排序的改进版。依然采用分治思想,先通过一趟排序将数据分割成两部分,其中一部分的所有数据要比另一部分的所有数据要小,然后再按此方法,对这两部分数据分别进行快速排序,整个过程可以用递归实现。
- 就是拿到一组数据,先找一个数,作为中间数(你可以直接拿序列中间的数,或者拿最后一个数,或者第一个数),然后依据此数,先排成两组,不用有序,就是比中间数大的,分为一组,比中间数小的,分为一组,中间数最后归哪组,随意,不归任何一组也可以。然后分别对这两组再进行快速排序(就像冒泡一样,每次把中间数冒出去)
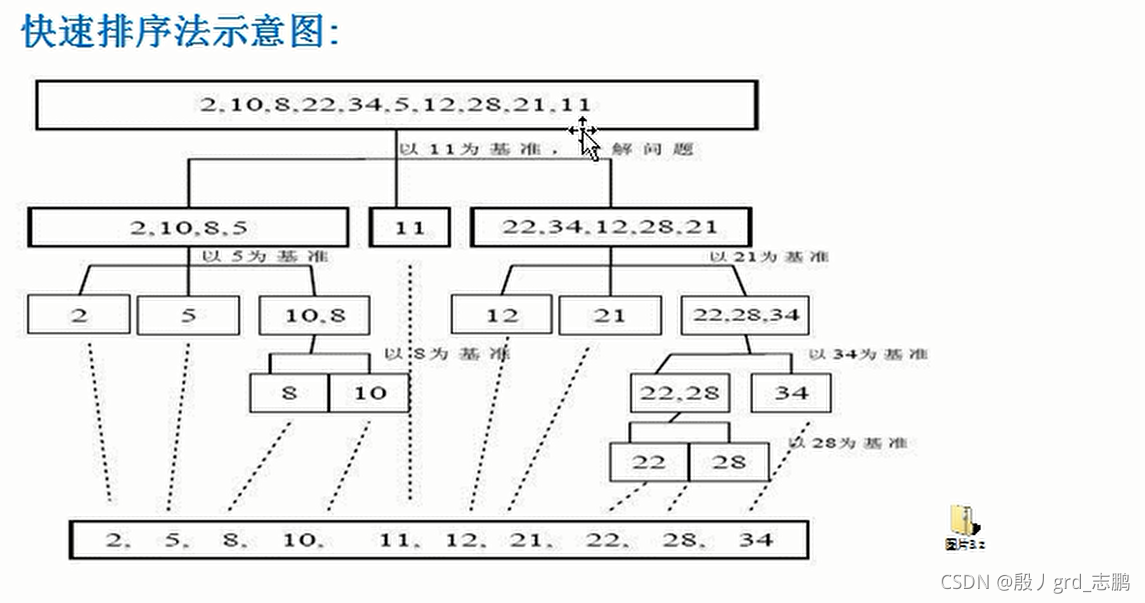
- 实现思路与运行效果
- 首先,我们选择中间数(基准数),我们这里指定序列的第一个数是基准数,将基准数保存。然后创建两个下标,分别位于序列的最左面和最右面,依次从右边找大于中间数的值,将其放在左边,左边找小于中间数的值,放在右边,最后,左边下标最后的位置,就是中间,将中间数放进去即可
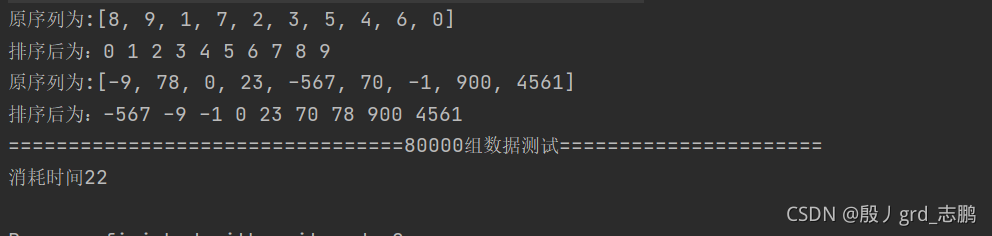
- 代码第一版
import java.util.Arrays;
import java.util.Date;
public class Test {
public static void main(String[] args) {
int arr[]= {8,9,1,7,2,3,5,4,6,0};
int arr1[]={-9,78,0,23,-567,70, -1,900, 4561};
System.out.println("原序列为:"+ Arrays.toString(arr));
quickSort(arr,0,arr.length-1);
print(arr);
System.out.println("原序列为:"+ Arrays.toString(arr1));
quickSort(arr1,0,arr1.length-1);
print(arr1);
System.out.println("=================================80000组数据测试======================");
int array[] = new int[80000];
for (int i = 0 ;i<80000;i++){
array[i] = (int) (Math.random()*800000);
}
long start = new Date().getTime();
quickSort(array,0,array.length-1);
long end = new Date().getTime();
System.out.println("消耗时间"+(end-start));
}
public static void quickSort(int[] a, int left, int right) {
if (left < right) {
int l = left;
int r = right;
int temp = a[l];
while (l < r) {
while(l < r && a[r] > temp)
r--;
if(l < r)
a[l++] = a[r];
while(l < r && a[l] < temp)
l++;
if(l < r)
a[r--] = a[l];
}
a[l] = temp;
quickSort(a, left, l-1);
quickSort(a, l+1,right);
}
}
public static void print(int arr[]){
System.out.print("排序后为:");
for(int i = 0;i<arr.length;i++){
System.out.print(arr[i]+" ");
}
System.out.println();
}
}
- 代码第二版
public void quickSort(int arr[]){
quickSort(arr,0,arr.length-1);
}
public void quickSort(int arr[],int low,int high){
if(low<high){
int pivotPosition = quickSortPartition(arr, low, high);
quickSort(arr,low,pivotPosition-1);
quickSort(arr,pivotPosition+1,high);
}
}
public int quickSortPartition(int arr[],int low,int high){
int pivot = arr[low];
while (low<high){
while(low<high && arr[high]>=pivot) --high;
arr[low] = arr[high];
while(low<high && arr[low]<=pivot) ++low;
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}